Spec2Pipeline for MRS (MIRI MRS CALSPEC2)¶
Spec2Pipeline
applies additional instrumental corrections and calibrations to count rate products that result in a fully calibrated individual exposure.
Official documentation for Spec2Pipeline
can be found here:
https://jwst-pipeline.readthedocs.io/en/latest/jwst/pipeline/calwebb_spec2.html
The Spec2Pipeline
for MRS comprises a linear series of steps. The steps in order are:
Step |
Description |
---|---|
|
attach WCS object to each science exposure |
|
subtract dedicated background exposures |
|
correct the exposure using the flat-field reference file |
|
if APT source type set to ‘UNKNOWN’, sets the ‘SRCTYPE’ keyword to ‘EXTENDED’ for MRS |
|
remove straylight for Channels 1/2 by interpolating across the inter-slice regions |
|
divide the data/error arrays by a fringe reference image |
|
apply flux (photometric) calibration |
|
produce 3D spectral cubes from the 2D detector image |
|
extract 1D spectrum from 3D cube |
Note that cube_build
and extract_1d
are not calibration steps but are contained in Spec2Pipeline
to provide users with cubes and 1D spectra from each exposure. Most users of these notebooks may not want to run these steps as they are only interested in the calibrated detector images and the cube_build
step in particular will dramatically processing time. Therefore, these steps will be skipped.
In this example, no background exposure will be provided so the background
step will not be run.
Input data¶
An example of running the file through the Spec2Pipeline
is now shown using a simple simulated fullband, 4-point dither observation of a point source (power law plus emission lines spectrum) with the MIRI MRS produced with MIRISim v2.3 and brought to level 2A using the Detector1Pipeline
.
Python¶
Start by importing what will be used and set the CRDS_CONTEXT
# imports
import os, glob, shutil
import numpy as np
from matplotlib.colors import LogNorm
import matplotlib.pyplot as plt
from subprocess import call
from jwst import datamodels
from jwst.associations.lib.member import Member
from jwst.associations.asn_from_list import asn_from_list
from jwst.associations.lib.rules_level2_base import DMSLevel2bBase
# set the CRDS_CONTEXT
os.environ["CRDS_CONTEXT"] = "jwst_0641.pmap"
Spec2Pipeline
can be run by passing indivual exposures or datamodels but in this sample case we produce an association file so all can be run through Spec2Pipeline
in a single call. It is assumed that the science files are in ‘MRS/SHORT’, ‘MRS/MEDIUM’, and ‘MRS/LONG’. The content of the association file is printed for inspection.
# load the science and background files to lists
my_science_files = glob.glob('MRS_science/*/*rate.fits')
my_science_files = sorted(my_science_files)
# setup an empty level 2 association structure
asn = asn_from_list(my_science_files, rule=DMSLevel2bBase)
asn.data['products'] = None
# set the association name
asn_name = 'my_point_source'
# set some metadata
asn['asn_pool'] = asn_name + '_pool'
asn['asn_type'] = 'spec2'
for n, sci in enumerate(my_science_files):
asn.new_product('{}_exposure{}'.format(asn_name, str(n+1)))
sci_member = Member({'expname': sci, 'exptype': 'science'})
new_members = asn.current_product['members']
new_members.append(sci_member)
# print the association and save to file
name, ser = asn.dump()
print(ser)
asn_file = asn_name + '_lvl2_asn.json'
with open(asn_file, 'w') as f:
f.write(ser)
{
"asn_type": "spec2",
"asn_rule": "DMSLevel2bBase",
"version_id": null,
"code_version": "0.17.1",
"degraded_status": "No known degraded exposures in association.",
"program": "noprogram",
"constraints": "No constraints",
"asn_id": "a3001",
"asn_pool": "my_point_source_pool",
"products": [
{
"name": "my_point_source_exposure1",
"members": [
{
"expname": "MRS_science/LONG/det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure2",
"members": [
{
"expname": "MRS_science/LONG/det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure3",
"members": [
{
"expname": "MRS_science/LONG/det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure4",
"members": [
{
"expname": "MRS_science/LONG/det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure5",
"members": [
{
"expname": "MRS_science/MEDIUM/det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure6",
"members": [
{
"expname": "MRS_science/MEDIUM/det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure7",
"members": [
{
"expname": "MRS_science/MEDIUM/det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure8",
"members": [
{
"expname": "MRS_science/MEDIUM/det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure9",
"members": [
{
"expname": "MRS_science/SHORT/det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure10",
"members": [
{
"expname": "MRS_science/SHORT/det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure11",
"members": [
{
"expname": "MRS_science/SHORT/det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure12",
"members": [
{
"expname": "MRS_science/SHORT/det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits",
"exptype": "science"
}
]
}
]
}
Import Spec2Pipeline and print the docstring to show some information
from jwst.pipeline import Spec2Pipeline
print(Spec2Pipeline.__doc__)
Spec2Pipeline: Processes JWST spectroscopic exposures from Level 2a to 2b.
Accepts a single exposure or an association as input.
Included steps are:
assign_wcs, background subtraction, NIRSpec MSA imprint subtraction,
NIRSpec MSA bad shutter flagging, 2-D subwindow extraction, flat field,
source type decision, straylight, fringe, pathloss, barshadow, photom,
resample_spec, cube_build, and extract_1d.
The association file is passed as input. The output level 2B files will be saved in my_output_dir
as _cal.fits
. The cubes and 1D spectra will also be saved.
Parameters used:
save_results
: boolean, optional, default=False
save the results to file
output_dir
: boolean, optional, default is the working directory
the location to save the output
steps
: dict, optional, default None
pass parameters to steps
# user specified
my_output_dir = 'MRS_science'
# the output directory should be created if it doesn't exist
if not os.path.exists(my_output_dir):
os.mkdir(my_output_dir)
# run the pipeline
Spec2Pipeline.call(asn_file, save_results=True, output_dir=my_output_dir,
steps={'cube_build': {'skip': True}, 'extract_1d': {'skip': True}})
2020-10-15 14:08:11,970 - CRDS - ERROR - Error determining best reference for 'pars-backgroundstep' = Unknown reference type 'pars-backgroundstep'
2020-10-15 14:08:12,029 - CRDS - ERROR - Error determining best reference for 'pars-assignwcsstep' = Unknown reference type 'pars-assignwcsstep'
2020-10-15 14:08:12,093 - CRDS - ERROR - Error determining best reference for 'pars-imprintstep' = Unknown reference type 'pars-imprintstep'
2020-10-15 14:08:12,152 - CRDS - ERROR - Error determining best reference for 'pars-msaflagopenstep' = Unknown reference type 'pars-msaflagopenstep'
2020-10-15 14:08:12,213 - CRDS - ERROR - Error determining best reference for 'pars-extract2dstep' = Unknown reference type 'pars-extract2dstep'
2020-10-15 14:08:12,276 - CRDS - ERROR - Error determining best reference for 'pars-flatfieldstep' = Unknown reference type 'pars-flatfieldstep'
2020-10-15 14:08:12,335 - CRDS - ERROR - Error determining best reference for 'pars-pathlossstep' = Unknown reference type 'pars-pathlossstep'
2020-10-15 14:08:12,395 - CRDS - ERROR - Error determining best reference for 'pars-barshadowstep' = Unknown reference type 'pars-barshadowstep'
2020-10-15 14:08:12,452 - CRDS - ERROR - Error determining best reference for 'pars-photomstep' = Unknown reference type 'pars-photomstep'
2020-10-15 14:08:12,524 - CRDS - ERROR - Error determining best reference for 'pars-masterbackgroundnrsslitsstep' = Unknown reference type 'pars-masterbackgroundnrsslitsstep'
2020-10-15 14:08:12,594 - CRDS - ERROR - Error determining best reference for 'pars-wavecorrstep' = Unknown reference type 'pars-wavecorrstep'
2020-10-15 14:08:12,664 - CRDS - ERROR - Error determining best reference for 'pars-flatfieldstep' = Unknown reference type 'pars-flatfieldstep'
2020-10-15 14:08:12,723 - CRDS - ERROR - Error determining best reference for 'pars-sourcetypestep' = Unknown reference type 'pars-sourcetypestep'
2020-10-15 14:08:12,780 - CRDS - ERROR - Error determining best reference for 'pars-straylightstep' = Unknown reference type 'pars-straylightstep'
2020-10-15 14:08:12,834 - CRDS - ERROR - Error determining best reference for 'pars-fringestep' = Unknown reference type 'pars-fringestep'
2020-10-15 14:08:12,890 - CRDS - ERROR - Error determining best reference for 'pars-pathlossstep' = Unknown reference type 'pars-pathlossstep'
2020-10-15 14:08:12,944 - CRDS - ERROR - Error determining best reference for 'pars-barshadowstep' = Unknown reference type 'pars-barshadowstep'
2020-10-15 14:08:13,003 - CRDS - ERROR - Error determining best reference for 'pars-photomstep' = Unknown reference type 'pars-photomstep'
2020-10-15 14:08:13,059 - CRDS - ERROR - Error determining best reference for 'pars-resamplespecstep' = Unknown reference type 'pars-resamplespecstep'
2020-10-15 14:08:13,115 - CRDS - ERROR - Error determining best reference for 'pars-cubebuildstep' = Unknown reference type 'pars-cubebuildstep'
2020-10-15 14:08:13,172 - CRDS - ERROR - Error determining best reference for 'pars-extract1dstep' = Unknown reference type 'pars-extract1dstep'
2020-10-15 14:08:13,228 - CRDS - ERROR - Error determining best reference for 'pars-spec2pipeline' = Unknown reference type 'pars-spec2pipeline'
2020-10-15 14:08:13,244 - stpipe.Spec2Pipeline - INFO - Spec2Pipeline instance created.
2020-10-15 14:08:13,246 - stpipe.Spec2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2020-10-15 14:08:13,248 - stpipe.Spec2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2020-10-15 14:08:13,250 - stpipe.Spec2Pipeline.imprint_subtract - INFO - ImprintStep instance created.
2020-10-15 14:08:13,252 - stpipe.Spec2Pipeline.msa_flagging - INFO - MSAFlagOpenStep instance created.
2020-10-15 14:08:13,254 - stpipe.Spec2Pipeline.extract_2d - INFO - Extract2dStep instance created.
2020-10-15 14:08:13,257 - stpipe.Spec2Pipeline.master_background - INFO - MasterBackgroundNrsSlitsStep instance created.
2020-10-15 14:08:13,259 - stpipe.Spec2Pipeline.master_background.flat_field - INFO - FlatFieldStep instance created.
2020-10-15 14:08:13,260 - stpipe.Spec2Pipeline.master_background.pathloss - INFO - PathLossStep instance created.
2020-10-15 14:08:13,263 - stpipe.Spec2Pipeline.master_background.barshadow - INFO - BarShadowStep instance created.
2020-10-15 14:08:13,264 - stpipe.Spec2Pipeline.master_background.photom - INFO - PhotomStep instance created.
2020-10-15 14:08:13,266 - stpipe.Spec2Pipeline.wavecorr - INFO - WavecorrStep instance created.
2020-10-15 14:08:13,268 - stpipe.Spec2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2020-10-15 14:08:13,270 - stpipe.Spec2Pipeline.srctype - INFO - SourceTypeStep instance created.
2020-10-15 14:08:13,271 - stpipe.Spec2Pipeline.straylight - INFO - StraylightStep instance created.
2020-10-15 14:08:13,273 - stpipe.Spec2Pipeline.fringe - INFO - FringeStep instance created.
2020-10-15 14:08:13,274 - stpipe.Spec2Pipeline.pathloss - INFO - PathLossStep instance created.
2020-10-15 14:08:13,276 - stpipe.Spec2Pipeline.barshadow - INFO - BarShadowStep instance created.
2020-10-15 14:08:13,279 - stpipe.Spec2Pipeline.photom - INFO - PhotomStep instance created.
2020-10-15 14:08:13,281 - stpipe.Spec2Pipeline.resample_spec - INFO - ResampleSpecStep instance created.
2020-10-15 14:08:13,284 - stpipe.Spec2Pipeline.cube_build - INFO - CubeBuildStep instance created.
2020-10-15 14:08:13,286 - stpipe.Spec2Pipeline.extract_1d - INFO - Extract1dStep instance created.
2020-10-15 14:08:13,762 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline running with args ('my_point_source_lvl2_asn.json',).
2020-10-15 14:08:13,779 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': 'MRS_science', 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'save_bsub': False, 'fail_on_exception': True, 'steps': {'bkg_subtract': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}, 'assign_wcs': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}, 'imprint_subtract': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': ''}, 'msa_flagging': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': ''}, 'extract_2d': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'slit_name': None, 'extract_orders': None, 'tsgrism_extract_height': None, 'wfss_extract_half_height': 5, 'grism_objects': None, 'mmag_extract': 99.0}, 'master_background': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'force_subtract': False, 'save_background': False, 'user_background': None, 'inverse': False, 'steps': {'flat_field': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}, 'pathloss': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}, 'barshadow': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}, 'photom': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}}}, 'wavecorr': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': ''}, 'flat_field': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}, 'srctype': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': ''}, 'straylight': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}, 'fringe': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': ''}, 'pathloss': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}, 'barshadow': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}, 'photom': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}, 'resample_spec': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'pixfrac': 1.0, 'kernel': 'square', 'fillval': 'INDEF', 'weight_type': 'exptime', 'single': False, 'blendheaders': True}, 'cube_build': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': None, 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'band', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}, 'extract_1d': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}}}
2020-10-15 14:08:13,848 - stpipe.Spec2Pipeline - INFO - Prefetching reference files for dataset: 'det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits' reftypes = ['area', 'barshadow', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'drizpars', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'fringe', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'msaoper', 'ote', 'pathloss', 'photom', 'regions', 'sflat', 'specwcs', 'wavecorr', 'wavelengthrange', 'wfssbkg']
2020-10-15 14:08:13,853 - stpipe.Spec2Pipeline - INFO - Prefetch for AREA reference file is 'N/A'.
2020-10-15 14:08:13,853 - stpipe.Spec2Pipeline - INFO - Prefetch for BARSHADOW reference file is 'N/A'.
2020-10-15 14:08:13,854 - stpipe.Spec2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2020-10-15 14:08:13,855 - stpipe.Spec2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2020-10-15 14:08:13,855 - stpipe.Spec2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2020-10-15 14:08:13,856 - stpipe.Spec2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2020-10-15 14:08:13,856 - stpipe.Spec2Pipeline - INFO - Prefetch for DISTORTION reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0032.asdf'.
2020-10-15 14:08:13,857 - stpipe.Spec2Pipeline - INFO - Prefetch for DRIZPARS reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_drizpars_0001.fits'.
2020-10-15 14:08:13,858 - stpipe.Spec2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2020-10-15 14:08:13,859 - stpipe.Spec2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is 'N/A'.
2020-10-15 14:08:13,859 - stpipe.Spec2Pipeline - INFO - Prefetch for FLAT reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_flat_0541.fits'.
2020-10-15 14:08:13,861 - stpipe.Spec2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2020-10-15 14:08:13,861 - stpipe.Spec2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2020-10-15 14:08:13,862 - stpipe.Spec2Pipeline - INFO - Prefetch for FRINGE reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0045.fits'.
2020-10-15 14:08:13,863 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2020-10-15 14:08:13,864 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2020-10-15 14:08:13,864 - stpipe.Spec2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2020-10-15 14:08:13,865 - stpipe.Spec2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2020-10-15 14:08:13,866 - stpipe.Spec2Pipeline - INFO - Prefetch for MSAOPER reference file is 'N/A'.
2020-10-15 14:08:13,866 - stpipe.Spec2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2020-10-15 14:08:13,867 - stpipe.Spec2Pipeline - INFO - Prefetch for PATHLOSS reference file is 'N/A'.
2020-10-15 14:08:13,867 - stpipe.Spec2Pipeline - INFO - Prefetch for PHOTOM reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0059.fits'.
2020-10-15 14:08:13,868 - stpipe.Spec2Pipeline - INFO - Prefetch for REGIONS reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0025.asdf'.
2020-10-15 14:08:13,869 - stpipe.Spec2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2020-10-15 14:08:13,870 - stpipe.Spec2Pipeline - INFO - Prefetch for SPECWCS reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0019.asdf'.
2020-10-15 14:08:13,870 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVECORR reference file is 'N/A'.
2020-10-15 14:08:13,871 - stpipe.Spec2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf'.
2020-10-15 14:08:13,871 - stpipe.Spec2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'N/A'.
2020-10-15 14:08:13,872 - stpipe.Spec2Pipeline - INFO - Starting calwebb_spec2 ...
2020-10-15 14:08:13,883 - stpipe.Spec2Pipeline - INFO - Processing product my_point_source_exposure1
2020-10-15 14:08:13,884 - stpipe.Spec2Pipeline - INFO - Working on input MRS_science/LONG/det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits ...
2020-10-15 14:08:14,359 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:14,360 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-15 14:08:17,595 - stpipe.Spec2Pipeline.assign_wcs - INFO - Created a MIRI mir_mrs pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0032.asdf', 'filteroffset': None, 'specwcs': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0019.asdf', 'regions': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0025.asdf', 'wavelengthrange': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf', 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-15 14:08:18,377 - stpipe.Spec2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.000000001 -0.001173020 359.999999995 -0.001173020 359.999999995 0.001089220 0.000000001 0.001089220
2020-10-15 14:08:18,377 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-15 14:08:18,382 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-15 14:08:18,871 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits>, []).
2020-10-15 14:08:18,873 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-15 14:08:18,873 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step skipped.
2020-10-15 14:08:18,874 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-15 14:08:19,291 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits>, []).
2020-10-15 14:08:19,293 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': None, 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:08:19,294 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2020-10-15 14:08:19,295 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract done
2020-10-15 14:08:19,722 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:19,723 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': None, 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:08:19,724 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2020-10-15 14:08:19,725 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging done
2020-10-15 14:08:20,175 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:20,177 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:08:20,186 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is MIR_MRS
2020-10-15 14:08:20,187 - stpipe.Spec2Pipeline.srctype - INFO - Input SRCTYAPT = None
2020-10-15 14:08:20,187 - stpipe.Spec2Pipeline.srctype - WARNING - SRCTYAPT keyword not found in input; using SRCTYPE instead
2020-10-15 14:08:20,188 - stpipe.Spec2Pipeline.srctype - INFO - Input source type is unknown; setting default SRCTYPE = EXTENDED
2020-10-15 14:08:20,189 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2020-10-15 14:08:20,602 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:20,603 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-15 14:08:20,667 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:08:21,621 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2020-10-15 14:08:22,062 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:22,063 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}
2020-10-15 14:08:22,072 - stpipe.Spec2Pipeline.straylight - WARNING - Straylight correction not defined for detector MIRIFULONG
2020-10-15 14:08:22,072 - stpipe.Spec2Pipeline.straylight - WARNING - Straylight step will be skipped
2020-10-15 14:08:22,962 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight done
2020-10-15 14:08:23,448 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:23,450 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:08:23,460 - stpipe.Spec2Pipeline.fringe - INFO - Using FRINGE reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0045.fits
2020-10-15 14:08:23,501 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:08:23,502 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:08:23,503 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:08:24,410 - stpipe.Spec2Pipeline.fringe - INFO - DQ values in the reference file NOT used to update the output DQ.
2020-10-15 14:08:24,412 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe done
2020-10-15 14:08:24,906 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:24,907 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:08:24,908 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2020-10-15 14:08:24,909 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss done
2020-10-15 14:08:25,365 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:25,367 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:08:25,368 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2020-10-15 14:08:25,369 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow done
2020-10-15 14:08:25,823 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:25,824 - stpipe.Spec2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:08:25,836 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0059.fits
2020-10-15 14:08:25,837 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2020-10-15 14:08:26,737 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-15 14:08:26,738 - stpipe.Spec2Pipeline.photom - INFO - detector: MIRIFULONG
2020-10-15 14:08:26,739 - stpipe.Spec2Pipeline.photom - INFO - exp_type: MIR_MRS
2020-10-15 14:08:26,739 - stpipe.Spec2Pipeline.photom - INFO - band: LONG
2020-10-15 14:08:26,802 - stpipe.Spec2Pipeline.photom - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:08:26,817 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2020-10-15 14:08:27,346 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure1_cal.fits>,).
2020-10-15 14:08:27,349 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': None, 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'multi', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}
2020-10-15 14:08:27,349 - stpipe.Spec2Pipeline.cube_build - INFO - Step skipped.
2020-10-15 14:08:27,350 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build done
2020-10-15 14:08:27,777 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure1_cal.fits>,).
2020-10-15 14:08:27,779 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': True, 'suffix': 'x1d', 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}
2020-10-15 14:08:27,780 - stpipe.Spec2Pipeline.extract_1d - INFO - Step skipped.
2020-10-15 14:08:27,781 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2020-10-15 14:08:27,782 - stpipe.Spec2Pipeline - INFO - Finished processing product my_point_source_exposure1
2020-10-15 14:08:27,783 - stpipe.Spec2Pipeline - INFO - Processing product my_point_source_exposure2
2020-10-15 14:08:27,783 - stpipe.Spec2Pipeline - INFO - Working on input MRS_science/LONG/det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits ...
2020-10-15 14:08:28,262 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:08:28,263 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-15 14:08:31,761 - stpipe.Spec2Pipeline.assign_wcs - INFO - Created a MIRI mir_mrs pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0029.asdf', 'filteroffset': None, 'specwcs': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0022.asdf', 'regions': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0028.asdf', 'wavelengthrange': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf', 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-15 14:08:33,138 - stpipe.Spec2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.000000001 -0.000731840 359.999999999 -0.000731840 359.999999999 0.000693132 0.000000001 0.000693132
2020-10-15 14:08:33,139 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-15 14:08:33,144 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-15 14:08:33,615 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits>, []).
2020-10-15 14:08:33,617 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'bkg_subtract', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-15 14:08:33,618 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step skipped.
2020-10-15 14:08:33,619 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-15 14:08:34,072 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits>, []).
2020-10-15 14:08:34,073 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'imprint_subtract', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:08:34,074 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2020-10-15 14:08:34,075 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract done
2020-10-15 14:08:34,529 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:08:34,530 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'msa_flagging', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:08:34,531 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2020-10-15 14:08:34,532 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging done
2020-10-15 14:08:34,981 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:08:34,982 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'srctype', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:08:34,992 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is MIR_MRS
2020-10-15 14:08:34,993 - stpipe.Spec2Pipeline.srctype - INFO - Input SRCTYAPT = None
2020-10-15 14:08:34,993 - stpipe.Spec2Pipeline.srctype - WARNING - SRCTYAPT keyword not found in input; using SRCTYPE instead
2020-10-15 14:08:34,994 - stpipe.Spec2Pipeline.srctype - INFO - Input source type is unknown; setting default SRCTYPE = EXTENDED
2020-10-15 14:08:34,995 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2020-10-15 14:08:35,447 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:08:35,449 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-15 14:08:35,511 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:08:36,755 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2020-10-15 14:08:37,253 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:08:37,255 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'straylight', 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}
2020-10-15 14:08:37,265 - stpipe.Spec2Pipeline.straylight - INFO - Using regions reference file /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0028.asdf
2020-10-15 14:08:37,284 - stpipe.Spec2Pipeline.straylight - INFO - Using 20% throughput threshhold.
2020-10-15 14:08:37,285 - stpipe.Spec2Pipeline.straylight - INFO - Modified Shepard weighting power 1.00
2020-10-15 14:08:37,285 - stpipe.Spec2Pipeline.straylight - INFO - Region of influence radius (pixels) 50.00
2020-10-15 14:08:43,979 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight done
2020-10-15 14:08:44,795 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:08:44,797 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'fringe', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:08:44,808 - stpipe.Spec2Pipeline.fringe - INFO - Using FRINGE reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0049.fits
2020-10-15 14:08:44,851 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:08:44,852 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:08:44,852 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:08:46,172 - stpipe.Spec2Pipeline.fringe - INFO - DQ values in the reference file NOT used to update the output DQ.
2020-10-15 14:08:46,173 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe done
2020-10-15 14:08:46,762 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:08:46,764 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'pathloss', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:08:46,765 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2020-10-15 14:08:46,766 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss done
2020-10-15 14:08:47,288 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:08:47,289 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'barshadow', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:08:47,290 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2020-10-15 14:08:47,291 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow done
2020-10-15 14:08:47,773 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:08:47,775 - stpipe.Spec2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:08:47,787 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0060.fits
2020-10-15 14:08:47,788 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2020-10-15 14:08:48,991 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-15 14:08:48,992 - stpipe.Spec2Pipeline.photom - INFO - detector: MIRIFUSHORT
2020-10-15 14:08:48,992 - stpipe.Spec2Pipeline.photom - INFO - exp_type: MIR_MRS
2020-10-15 14:08:48,993 - stpipe.Spec2Pipeline.photom - INFO - band: LONG
2020-10-15 14:08:49,055 - stpipe.Spec2Pipeline.photom - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:08:49,069 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2020-10-15 14:08:49,727 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure2_cal.fits>,).
2020-10-15 14:08:49,729 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'cube_build', 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'multi', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}
2020-10-15 14:08:49,730 - stpipe.Spec2Pipeline.cube_build - INFO - Step skipped.
2020-10-15 14:08:49,731 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build done
2020-10-15 14:08:50,308 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure2_cal.fits>,).
2020-10-15 14:08:50,311 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': True, 'suffix': 'x1d', 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}
2020-10-15 14:08:50,313 - stpipe.Spec2Pipeline.extract_1d - INFO - Step skipped.
2020-10-15 14:08:50,317 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2020-10-15 14:08:50,319 - stpipe.Spec2Pipeline - INFO - Finished processing product my_point_source_exposure2
2020-10-15 14:08:50,320 - stpipe.Spec2Pipeline - INFO - Processing product my_point_source_exposure3
2020-10-15 14:08:50,320 - stpipe.Spec2Pipeline - INFO - Working on input MRS_science/LONG/det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits ...
2020-10-15 14:08:50,908 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:50,910 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-15 14:08:53,877 - stpipe.Spec2Pipeline.assign_wcs - INFO - Created a MIRI mir_mrs pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0032.asdf', 'filteroffset': None, 'specwcs': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0019.asdf', 'regions': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0025.asdf', 'wavelengthrange': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf', 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-15 14:08:54,975 - stpipe.Spec2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.000000004 -0.001362535 359.999999998 -0.001362535 359.999999998 0.000899705 0.000000004 0.000899705
2020-10-15 14:08:54,976 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-15 14:08:54,982 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-15 14:08:55,721 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits>, []).
2020-10-15 14:08:55,724 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'bkg_subtract', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-15 14:08:55,726 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step skipped.
2020-10-15 14:08:55,728 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-15 14:08:56,410 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits>, []).
2020-10-15 14:08:56,412 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'imprint_subtract', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:08:56,412 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2020-10-15 14:08:56,413 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract done
2020-10-15 14:08:56,930 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:56,932 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'msa_flagging', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:08:56,932 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2020-10-15 14:08:56,933 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging done
2020-10-15 14:08:57,503 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:57,505 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'srctype', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:08:57,516 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is MIR_MRS
2020-10-15 14:08:57,517 - stpipe.Spec2Pipeline.srctype - INFO - Input SRCTYAPT = None
2020-10-15 14:08:57,518 - stpipe.Spec2Pipeline.srctype - WARNING - SRCTYAPT keyword not found in input; using SRCTYPE instead
2020-10-15 14:08:57,519 - stpipe.Spec2Pipeline.srctype - INFO - Input source type is unknown; setting default SRCTYPE = EXTENDED
2020-10-15 14:08:57,520 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2020-10-15 14:08:58,047 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:58,049 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-15 14:08:58,126 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:08:59,133 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2020-10-15 14:08:59,683 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:08:59,685 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'straylight', 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}
2020-10-15 14:08:59,694 - stpipe.Spec2Pipeline.straylight - WARNING - Straylight correction not defined for detector MIRIFULONG
2020-10-15 14:08:59,695 - stpipe.Spec2Pipeline.straylight - WARNING - Straylight step will be skipped
2020-10-15 14:09:00,629 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight done
2020-10-15 14:09:01,255 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:09:01,257 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'fringe', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:09:01,269 - stpipe.Spec2Pipeline.fringe - INFO - Using FRINGE reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0045.fits
2020-10-15 14:09:01,321 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:01,322 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:01,322 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:02,366 - stpipe.Spec2Pipeline.fringe - INFO - DQ values in the reference file NOT used to update the output DQ.
2020-10-15 14:09:02,368 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe done
2020-10-15 14:09:02,950 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:09:02,952 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'pathloss', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:09:02,953 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2020-10-15 14:09:02,954 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss done
2020-10-15 14:09:03,477 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:09:03,478 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'barshadow', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:09:03,479 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2020-10-15 14:09:03,480 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow done
2020-10-15 14:09:03,989 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits>,).
2020-10-15 14:09:03,991 - stpipe.Spec2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:09:04,004 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0059.fits
2020-10-15 14:09:04,005 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2020-10-15 14:09:04,920 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-15 14:09:04,920 - stpipe.Spec2Pipeline.photom - INFO - detector: MIRIFULONG
2020-10-15 14:09:04,921 - stpipe.Spec2Pipeline.photom - INFO - exp_type: MIR_MRS
2020-10-15 14:09:04,921 - stpipe.Spec2Pipeline.photom - INFO - band: LONG
2020-10-15 14:09:04,983 - stpipe.Spec2Pipeline.photom - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:04,997 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2020-10-15 14:09:05,595 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure3_cal.fits>,).
2020-10-15 14:09:05,597 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'cube_build', 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'multi', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}
2020-10-15 14:09:05,598 - stpipe.Spec2Pipeline.cube_build - INFO - Step skipped.
2020-10-15 14:09:05,599 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build done
2020-10-15 14:09:06,086 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure3_cal.fits>,).
2020-10-15 14:09:06,088 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': True, 'suffix': 'x1d', 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}
2020-10-15 14:09:06,088 - stpipe.Spec2Pipeline.extract_1d - INFO - Step skipped.
2020-10-15 14:09:06,090 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2020-10-15 14:09:06,090 - stpipe.Spec2Pipeline - INFO - Finished processing product my_point_source_exposure3
2020-10-15 14:09:06,091 - stpipe.Spec2Pipeline - INFO - Processing product my_point_source_exposure4
2020-10-15 14:09:06,091 - stpipe.Spec2Pipeline - INFO - Working on input MRS_science/LONG/det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits ...
2020-10-15 14:09:06,625 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:09:06,627 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-15 14:09:10,253 - stpipe.Spec2Pipeline.assign_wcs - INFO - Created a MIRI mir_mrs pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0029.asdf', 'filteroffset': None, 'specwcs': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0022.asdf', 'regions': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0028.asdf', 'wavelengthrange': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf', 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-15 14:09:11,860 - stpipe.Spec2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.000000001 -0.000921354 359.999999997 -0.000921354 359.999999997 0.000503617 0.000000001 0.000503617
2020-10-15 14:09:11,861 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-15 14:09:11,867 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-15 14:09:12,586 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits>, []).
2020-10-15 14:09:12,587 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'bkg_subtract', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-15 14:09:12,588 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step skipped.
2020-10-15 14:09:12,589 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-15 14:09:13,230 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits>, []).
2020-10-15 14:09:13,231 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'imprint_subtract', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:09:13,233 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2020-10-15 14:09:13,234 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract done
2020-10-15 14:09:13,798 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:09:13,800 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'msa_flagging', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:09:13,800 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2020-10-15 14:09:13,801 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging done
2020-10-15 14:09:14,329 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:09:14,330 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'srctype', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:09:14,341 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is MIR_MRS
2020-10-15 14:09:14,342 - stpipe.Spec2Pipeline.srctype - INFO - Input SRCTYAPT = None
2020-10-15 14:09:14,342 - stpipe.Spec2Pipeline.srctype - WARNING - SRCTYAPT keyword not found in input; using SRCTYPE instead
2020-10-15 14:09:14,343 - stpipe.Spec2Pipeline.srctype - INFO - Input source type is unknown; setting default SRCTYPE = EXTENDED
2020-10-15 14:09:14,344 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2020-10-15 14:09:14,921 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:09:14,922 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-15 14:09:14,992 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:16,235 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2020-10-15 14:09:16,838 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:09:16,839 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'straylight', 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}
2020-10-15 14:09:16,849 - stpipe.Spec2Pipeline.straylight - INFO - Using regions reference file /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0028.asdf
2020-10-15 14:09:16,874 - stpipe.Spec2Pipeline.straylight - INFO - Using 20% throughput threshhold.
2020-10-15 14:09:16,875 - stpipe.Spec2Pipeline.straylight - INFO - Modified Shepard weighting power 1.00
2020-10-15 14:09:16,875 - stpipe.Spec2Pipeline.straylight - INFO - Region of influence radius (pixels) 50.00
2020-10-15 14:09:22,740 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight done
2020-10-15 14:09:23,345 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:09:23,347 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'fringe', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:09:23,358 - stpipe.Spec2Pipeline.fringe - INFO - Using FRINGE reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0049.fits
2020-10-15 14:09:23,400 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:23,401 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:23,402 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:24,595 - stpipe.Spec2Pipeline.fringe - INFO - DQ values in the reference file NOT used to update the output DQ.
2020-10-15 14:09:24,597 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe done
2020-10-15 14:09:25,209 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:09:25,211 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'pathloss', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:09:25,213 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2020-10-15 14:09:25,214 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss done
2020-10-15 14:09:25,808 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:09:25,810 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'barshadow', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:09:25,811 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2020-10-15 14:09:25,813 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow done
2020-10-15 14:09:26,391 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits>,).
2020-10-15 14:09:26,393 - stpipe.Spec2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:09:26,407 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0060.fits
2020-10-15 14:09:26,407 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2020-10-15 14:09:27,620 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-15 14:09:27,621 - stpipe.Spec2Pipeline.photom - INFO - detector: MIRIFUSHORT
2020-10-15 14:09:27,621 - stpipe.Spec2Pipeline.photom - INFO - exp_type: MIR_MRS
2020-10-15 14:09:27,622 - stpipe.Spec2Pipeline.photom - INFO - band: LONG
2020-10-15 14:09:27,679 - stpipe.Spec2Pipeline.photom - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:27,693 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2020-10-15 14:09:28,344 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure4_cal.fits>,).
2020-10-15 14:09:28,346 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'cube_build', 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'multi', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}
2020-10-15 14:09:28,347 - stpipe.Spec2Pipeline.cube_build - INFO - Step skipped.
2020-10-15 14:09:28,348 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build done
2020-10-15 14:09:28,865 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure4_cal.fits>,).
2020-10-15 14:09:28,867 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': True, 'suffix': 'x1d', 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}
2020-10-15 14:09:28,868 - stpipe.Spec2Pipeline.extract_1d - INFO - Step skipped.
2020-10-15 14:09:28,869 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2020-10-15 14:09:28,870 - stpipe.Spec2Pipeline - INFO - Finished processing product my_point_source_exposure4
2020-10-15 14:09:28,870 - stpipe.Spec2Pipeline - INFO - Processing product my_point_source_exposure5
2020-10-15 14:09:28,871 - stpipe.Spec2Pipeline - INFO - Working on input MRS_science/MEDIUM/det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits ...
2020-10-15 14:09:29,443 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:29,445 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-15 14:09:32,084 - stpipe.Spec2Pipeline.assign_wcs - INFO - Created a MIRI mir_mrs pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0033.asdf', 'filteroffset': None, 'specwcs': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0023.asdf', 'regions': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0030.asdf', 'wavelengthrange': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf', 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-15 14:09:32,858 - stpipe.Spec2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.000000000 -0.001185998 359.999999999 -0.001185998 359.999999999 0.001071963 0.000000000 0.001071963
2020-10-15 14:09:32,858 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-15 14:09:32,863 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-15 14:09:33,475 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits>, []).
2020-10-15 14:09:33,476 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'bkg_subtract', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-15 14:09:33,477 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step skipped.
2020-10-15 14:09:33,478 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-15 14:09:34,009 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits>, []).
2020-10-15 14:09:34,010 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'imprint_subtract', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:09:34,012 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2020-10-15 14:09:34,013 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract done
2020-10-15 14:09:34,534 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:34,536 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'msa_flagging', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:09:34,536 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2020-10-15 14:09:34,537 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging done
2020-10-15 14:09:35,061 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:35,063 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'srctype', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:09:35,072 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is MIR_MRS
2020-10-15 14:09:35,073 - stpipe.Spec2Pipeline.srctype - INFO - Input SRCTYAPT = None
2020-10-15 14:09:35,073 - stpipe.Spec2Pipeline.srctype - WARNING - SRCTYAPT keyword not found in input; using SRCTYPE instead
2020-10-15 14:09:35,074 - stpipe.Spec2Pipeline.srctype - INFO - Input source type is unknown; setting default SRCTYPE = EXTENDED
2020-10-15 14:09:35,076 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2020-10-15 14:09:35,615 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:35,617 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-15 14:09:35,682 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:36,649 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2020-10-15 14:09:37,233 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:37,234 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'straylight', 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}
2020-10-15 14:09:37,243 - stpipe.Spec2Pipeline.straylight - WARNING - Straylight correction not defined for detector MIRIFULONG
2020-10-15 14:09:37,243 - stpipe.Spec2Pipeline.straylight - WARNING - Straylight step will be skipped
2020-10-15 14:09:38,141 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight done
2020-10-15 14:09:38,801 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:38,803 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'fringe', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:09:38,814 - stpipe.Spec2Pipeline.fringe - INFO - Using FRINGE reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0044.fits
2020-10-15 14:09:38,856 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:38,857 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:38,857 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:39,911 - stpipe.Spec2Pipeline.fringe - INFO - DQ values in the reference file NOT used to update the output DQ.
2020-10-15 14:09:39,913 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe done
2020-10-15 14:09:40,641 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:40,644 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'pathloss', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:09:40,645 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2020-10-15 14:09:40,647 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss done
2020-10-15 14:09:41,342 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:41,343 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'barshadow', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:09:41,344 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2020-10-15 14:09:41,345 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow done
2020-10-15 14:09:41,919 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:41,921 - stpipe.Spec2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:09:41,933 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0049.fits
2020-10-15 14:09:41,934 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2020-10-15 14:09:42,830 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-15 14:09:42,830 - stpipe.Spec2Pipeline.photom - INFO - detector: MIRIFULONG
2020-10-15 14:09:42,831 - stpipe.Spec2Pipeline.photom - INFO - exp_type: MIR_MRS
2020-10-15 14:09:42,831 - stpipe.Spec2Pipeline.photom - INFO - band: MEDIUM
2020-10-15 14:09:42,894 - stpipe.Spec2Pipeline.photom - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:42,910 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2020-10-15 14:09:43,565 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure5_cal.fits>,).
2020-10-15 14:09:43,568 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'cube_build', 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'multi', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}
2020-10-15 14:09:43,568 - stpipe.Spec2Pipeline.cube_build - INFO - Step skipped.
2020-10-15 14:09:43,570 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build done
2020-10-15 14:09:44,112 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure5_cal.fits>,).
2020-10-15 14:09:44,114 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': True, 'suffix': 'x1d', 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}
2020-10-15 14:09:44,115 - stpipe.Spec2Pipeline.extract_1d - INFO - Step skipped.
2020-10-15 14:09:44,116 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2020-10-15 14:09:44,116 - stpipe.Spec2Pipeline - INFO - Finished processing product my_point_source_exposure5
2020-10-15 14:09:44,117 - stpipe.Spec2Pipeline - INFO - Processing product my_point_source_exposure6
2020-10-15 14:09:44,117 - stpipe.Spec2Pipeline - INFO - Working on input MRS_science/MEDIUM/det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits ...
2020-10-15 14:09:44,757 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:44,758 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-15 14:09:48,473 - stpipe.Spec2Pipeline.assign_wcs - INFO - Created a MIRI mir_mrs pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0030.asdf', 'filteroffset': None, 'specwcs': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0021.asdf', 'regions': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0026.asdf', 'wavelengthrange': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf', 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-15 14:09:49,377 - stpipe.Spec2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.000000002 -0.000746626 359.999999999 -0.000746626 359.999999999 0.000639623 0.000000002 0.000639623
2020-10-15 14:09:49,378 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-15 14:09:49,384 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-15 14:09:50,090 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits>, []).
2020-10-15 14:09:50,092 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'bkg_subtract', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-15 14:09:50,093 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step skipped.
2020-10-15 14:09:50,094 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-15 14:09:50,765 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits>, []).
2020-10-15 14:09:50,766 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'imprint_subtract', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:09:50,767 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2020-10-15 14:09:50,768 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract done
2020-10-15 14:09:51,384 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:51,386 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'msa_flagging', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:09:51,386 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2020-10-15 14:09:51,388 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging done
2020-10-15 14:09:51,997 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:51,998 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'srctype', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:09:52,008 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is MIR_MRS
2020-10-15 14:09:52,009 - stpipe.Spec2Pipeline.srctype - INFO - Input SRCTYAPT = None
2020-10-15 14:09:52,010 - stpipe.Spec2Pipeline.srctype - WARNING - SRCTYAPT keyword not found in input; using SRCTYPE instead
2020-10-15 14:09:52,010 - stpipe.Spec2Pipeline.srctype - INFO - Input source type is unknown; setting default SRCTYPE = EXTENDED
2020-10-15 14:09:52,011 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2020-10-15 14:09:52,609 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:52,611 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-15 14:09:52,675 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:09:53,950 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2020-10-15 14:09:54,600 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:09:54,602 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'straylight', 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}
2020-10-15 14:09:54,612 - stpipe.Spec2Pipeline.straylight - INFO - Using regions reference file /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0026.asdf
2020-10-15 14:09:54,636 - stpipe.Spec2Pipeline.straylight - INFO - Using 20% throughput threshhold.
2020-10-15 14:09:54,637 - stpipe.Spec2Pipeline.straylight - INFO - Modified Shepard weighting power 1.00
2020-10-15 14:09:54,637 - stpipe.Spec2Pipeline.straylight - INFO - Region of influence radius (pixels) 50.00
2020-10-15 14:10:00,695 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight done
2020-10-15 14:10:01,437 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:01,439 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'fringe', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:10:01,450 - stpipe.Spec2Pipeline.fringe - INFO - Using FRINGE reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0034.fits
2020-10-15 14:10:01,497 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:01,498 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:01,498 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:02,740 - stpipe.Spec2Pipeline.fringe - INFO - DQ values in the reference file NOT used to update the output DQ.
2020-10-15 14:10:02,742 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe done
2020-10-15 14:10:03,561 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:03,563 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'pathloss', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:10:03,563 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2020-10-15 14:10:03,565 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss done
2020-10-15 14:10:04,261 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:04,263 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'barshadow', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:10:04,264 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2020-10-15 14:10:04,265 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow done
2020-10-15 14:10:04,939 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:04,941 - stpipe.Spec2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:10:04,954 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0064.fits
2020-10-15 14:10:04,955 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2020-10-15 14:10:06,220 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-15 14:10:06,221 - stpipe.Spec2Pipeline.photom - INFO - detector: MIRIFUSHORT
2020-10-15 14:10:06,221 - stpipe.Spec2Pipeline.photom - INFO - exp_type: MIR_MRS
2020-10-15 14:10:06,222 - stpipe.Spec2Pipeline.photom - INFO - band: MEDIUM
2020-10-15 14:10:06,290 - stpipe.Spec2Pipeline.photom - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:06,307 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2020-10-15 14:10:07,064 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure6_cal.fits>,).
2020-10-15 14:10:07,067 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'cube_build', 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'multi', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}
2020-10-15 14:10:07,068 - stpipe.Spec2Pipeline.cube_build - INFO - Step skipped.
2020-10-15 14:10:07,069 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build done
2020-10-15 14:10:07,699 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure6_cal.fits>,).
2020-10-15 14:10:07,702 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': True, 'suffix': 'x1d', 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}
2020-10-15 14:10:07,703 - stpipe.Spec2Pipeline.extract_1d - INFO - Step skipped.
2020-10-15 14:10:07,704 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2020-10-15 14:10:07,706 - stpipe.Spec2Pipeline - INFO - Finished processing product my_point_source_exposure6
2020-10-15 14:10:07,707 - stpipe.Spec2Pipeline - INFO - Processing product my_point_source_exposure7
2020-10-15 14:10:07,708 - stpipe.Spec2Pipeline - INFO - Working on input MRS_science/MEDIUM/det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits ...
2020-10-15 14:10:08,413 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:08,414 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-15 14:10:11,211 - stpipe.Spec2Pipeline.assign_wcs - INFO - Created a MIRI mir_mrs pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0033.asdf', 'filteroffset': None, 'specwcs': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0023.asdf', 'regions': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0030.asdf', 'wavelengthrange': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf', 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-15 14:10:11,991 - stpipe.Spec2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.000000006 -0.001375513 360.000000000 -0.001375513 360.000000000 0.000882449 0.000000006 0.000882449
2020-10-15 14:10:11,991 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-15 14:10:11,997 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-15 14:10:12,708 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits>, []).
2020-10-15 14:10:12,709 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'bkg_subtract', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-15 14:10:12,710 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step skipped.
2020-10-15 14:10:12,711 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-15 14:10:13,344 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits>, []).
2020-10-15 14:10:13,346 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'imprint_subtract', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:10:13,346 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2020-10-15 14:10:13,348 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract done
2020-10-15 14:10:13,979 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:13,980 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'msa_flagging', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:10:13,981 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2020-10-15 14:10:13,982 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging done
2020-10-15 14:10:14,613 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:14,614 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'srctype', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:10:14,625 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is MIR_MRS
2020-10-15 14:10:14,626 - stpipe.Spec2Pipeline.srctype - INFO - Input SRCTYAPT = None
2020-10-15 14:10:14,626 - stpipe.Spec2Pipeline.srctype - WARNING - SRCTYAPT keyword not found in input; using SRCTYPE instead
2020-10-15 14:10:14,627 - stpipe.Spec2Pipeline.srctype - INFO - Input source type is unknown; setting default SRCTYPE = EXTENDED
2020-10-15 14:10:14,629 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2020-10-15 14:10:15,260 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:15,262 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-15 14:10:15,328 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:16,321 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2020-10-15 14:10:16,976 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:16,977 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'straylight', 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}
2020-10-15 14:10:16,986 - stpipe.Spec2Pipeline.straylight - WARNING - Straylight correction not defined for detector MIRIFULONG
2020-10-15 14:10:16,987 - stpipe.Spec2Pipeline.straylight - WARNING - Straylight step will be skipped
2020-10-15 14:10:17,937 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight done
2020-10-15 14:10:18,641 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:18,643 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'fringe', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:10:18,654 - stpipe.Spec2Pipeline.fringe - INFO - Using FRINGE reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0044.fits
2020-10-15 14:10:18,694 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:18,695 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:18,695 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:19,666 - stpipe.Spec2Pipeline.fringe - INFO - DQ values in the reference file NOT used to update the output DQ.
2020-10-15 14:10:19,668 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe done
2020-10-15 14:10:20,373 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:20,375 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'pathloss', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:10:20,376 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2020-10-15 14:10:20,377 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss done
2020-10-15 14:10:21,097 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:21,099 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'barshadow', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:10:21,100 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2020-10-15 14:10:21,101 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow done
2020-10-15 14:10:21,753 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:21,754 - stpipe.Spec2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:10:21,767 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0049.fits
2020-10-15 14:10:21,768 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2020-10-15 14:10:22,676 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-15 14:10:22,677 - stpipe.Spec2Pipeline.photom - INFO - detector: MIRIFULONG
2020-10-15 14:10:22,677 - stpipe.Spec2Pipeline.photom - INFO - exp_type: MIR_MRS
2020-10-15 14:10:22,678 - stpipe.Spec2Pipeline.photom - INFO - band: MEDIUM
2020-10-15 14:10:22,743 - stpipe.Spec2Pipeline.photom - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:22,758 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2020-10-15 14:10:23,494 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure7_cal.fits>,).
2020-10-15 14:10:23,497 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'cube_build', 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'multi', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}
2020-10-15 14:10:23,497 - stpipe.Spec2Pipeline.cube_build - INFO - Step skipped.
2020-10-15 14:10:23,498 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build done
2020-10-15 14:10:24,104 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure7_cal.fits>,).
2020-10-15 14:10:24,105 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': True, 'suffix': 'x1d', 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}
2020-10-15 14:10:24,106 - stpipe.Spec2Pipeline.extract_1d - INFO - Step skipped.
2020-10-15 14:10:24,107 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2020-10-15 14:10:24,108 - stpipe.Spec2Pipeline - INFO - Finished processing product my_point_source_exposure7
2020-10-15 14:10:24,108 - stpipe.Spec2Pipeline - INFO - Processing product my_point_source_exposure8
2020-10-15 14:10:24,109 - stpipe.Spec2Pipeline - INFO - Working on input MRS_science/MEDIUM/det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits ...
2020-10-15 14:10:24,865 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:24,866 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-15 14:10:28,639 - stpipe.Spec2Pipeline.assign_wcs - INFO - Created a MIRI mir_mrs pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0030.asdf', 'filteroffset': None, 'specwcs': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0021.asdf', 'regions': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0026.asdf', 'wavelengthrange': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf', 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-15 14:10:29,539 - stpipe.Spec2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.000000002 -0.000936140 359.999999999 -0.000936140 359.999999999 0.000450109 0.000000002 0.000450109
2020-10-15 14:10:29,540 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-15 14:10:29,545 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-15 14:10:30,290 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits>, []).
2020-10-15 14:10:30,292 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'bkg_subtract', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-15 14:10:30,292 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step skipped.
2020-10-15 14:10:30,293 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-15 14:10:30,957 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits>, []).
2020-10-15 14:10:30,959 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'imprint_subtract', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:10:30,959 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2020-10-15 14:10:30,961 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract done
2020-10-15 14:10:31,684 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:31,689 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'msa_flagging', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:10:31,698 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2020-10-15 14:10:31,700 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging done
2020-10-15 14:10:32,578 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:32,580 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'srctype', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:10:32,589 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is MIR_MRS
2020-10-15 14:10:32,590 - stpipe.Spec2Pipeline.srctype - INFO - Input SRCTYAPT = None
2020-10-15 14:10:32,590 - stpipe.Spec2Pipeline.srctype - WARNING - SRCTYAPT keyword not found in input; using SRCTYPE instead
2020-10-15 14:10:32,591 - stpipe.Spec2Pipeline.srctype - INFO - Input source type is unknown; setting default SRCTYPE = EXTENDED
2020-10-15 14:10:32,593 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2020-10-15 14:10:33,264 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:33,266 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-15 14:10:33,329 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:34,636 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2020-10-15 14:10:35,419 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:35,422 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'straylight', 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}
2020-10-15 14:10:35,433 - stpipe.Spec2Pipeline.straylight - INFO - Using regions reference file /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0026.asdf
2020-10-15 14:10:35,455 - stpipe.Spec2Pipeline.straylight - INFO - Using 20% throughput threshhold.
2020-10-15 14:10:35,456 - stpipe.Spec2Pipeline.straylight - INFO - Modified Shepard weighting power 1.00
2020-10-15 14:10:35,457 - stpipe.Spec2Pipeline.straylight - INFO - Region of influence radius (pixels) 50.00
2020-10-15 14:10:41,460 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight done
2020-10-15 14:10:42,247 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:42,248 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'fringe', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:10:42,260 - stpipe.Spec2Pipeline.fringe - INFO - Using FRINGE reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0034.fits
2020-10-15 14:10:42,300 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:42,301 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:42,301 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:43,574 - stpipe.Spec2Pipeline.fringe - INFO - DQ values in the reference file NOT used to update the output DQ.
2020-10-15 14:10:43,576 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe done
2020-10-15 14:10:44,364 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:44,366 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'pathloss', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:10:44,366 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2020-10-15 14:10:44,367 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss done
2020-10-15 14:10:45,089 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:45,091 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'barshadow', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:10:45,092 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2020-10-15 14:10:45,093 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow done
2020-10-15 14:10:45,848 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits>,).
2020-10-15 14:10:45,850 - stpipe.Spec2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:10:45,863 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0064.fits
2020-10-15 14:10:45,864 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2020-10-15 14:10:47,159 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-15 14:10:47,160 - stpipe.Spec2Pipeline.photom - INFO - detector: MIRIFUSHORT
2020-10-15 14:10:47,160 - stpipe.Spec2Pipeline.photom - INFO - exp_type: MIR_MRS
2020-10-15 14:10:47,161 - stpipe.Spec2Pipeline.photom - INFO - band: MEDIUM
2020-10-15 14:10:47,219 - stpipe.Spec2Pipeline.photom - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:47,234 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2020-10-15 14:10:48,073 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure8_cal.fits>,).
2020-10-15 14:10:48,076 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'cube_build', 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'multi', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}
2020-10-15 14:10:48,076 - stpipe.Spec2Pipeline.cube_build - INFO - Step skipped.
2020-10-15 14:10:48,077 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build done
2020-10-15 14:10:48,763 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure8_cal.fits>,).
2020-10-15 14:10:48,765 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': True, 'suffix': 'x1d', 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}
2020-10-15 14:10:48,766 - stpipe.Spec2Pipeline.extract_1d - INFO - Step skipped.
2020-10-15 14:10:48,767 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2020-10-15 14:10:48,767 - stpipe.Spec2Pipeline - INFO - Finished processing product my_point_source_exposure8
2020-10-15 14:10:48,768 - stpipe.Spec2Pipeline - INFO - Processing product my_point_source_exposure9
2020-10-15 14:10:48,769 - stpipe.Spec2Pipeline - INFO - Working on input MRS_science/SHORT/det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits ...
2020-10-15 14:10:49,510 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:10:49,511 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-15 14:10:52,415 - stpipe.Spec2Pipeline.assign_wcs - INFO - Created a MIRI mir_mrs pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0034.asdf', 'filteroffset': None, 'specwcs': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0020.asdf', 'regions': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0029.asdf', 'wavelengthrange': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf', 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-15 14:10:53,217 - stpipe.Spec2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.000000001 -0.001205750 359.999999999 -0.001205750 359.999999999 0.001056315 0.000000001 0.001056315
2020-10-15 14:10:53,218 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-15 14:10:53,223 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-15 14:10:54,006 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits>, []).
2020-10-15 14:10:54,007 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'bkg_subtract', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-15 14:10:54,008 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step skipped.
2020-10-15 14:10:54,009 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-15 14:10:54,829 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits>, []).
2020-10-15 14:10:54,831 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'imprint_subtract', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:10:54,832 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2020-10-15 14:10:54,833 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract done
2020-10-15 14:10:55,544 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:10:55,546 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'msa_flagging', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:10:55,546 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2020-10-15 14:10:55,548 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging done
2020-10-15 14:10:56,249 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:10:56,250 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'srctype', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:10:56,260 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is MIR_MRS
2020-10-15 14:10:56,261 - stpipe.Spec2Pipeline.srctype - INFO - Input SRCTYAPT = None
2020-10-15 14:10:56,261 - stpipe.Spec2Pipeline.srctype - WARNING - SRCTYAPT keyword not found in input; using SRCTYPE instead
2020-10-15 14:10:56,262 - stpipe.Spec2Pipeline.srctype - INFO - Input source type is unknown; setting default SRCTYPE = EXTENDED
2020-10-15 14:10:56,263 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2020-10-15 14:10:57,007 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:10:57,010 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-15 14:10:57,084 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:10:58,161 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2020-10-15 14:10:58,873 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:10:58,875 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'straylight', 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}
2020-10-15 14:10:58,883 - stpipe.Spec2Pipeline.straylight - WARNING - Straylight correction not defined for detector MIRIFULONG
2020-10-15 14:10:58,884 - stpipe.Spec2Pipeline.straylight - WARNING - Straylight step will be skipped
2020-10-15 14:10:59,798 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight done
2020-10-15 14:11:00,599 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:00,601 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'fringe', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:11:00,611 - stpipe.Spec2Pipeline.fringe - INFO - Using FRINGE reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0042.fits
2020-10-15 14:11:00,654 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:00,655 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:00,656 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:01,602 - stpipe.Spec2Pipeline.fringe - INFO - DQ values in the reference file NOT used to update the output DQ.
2020-10-15 14:11:01,604 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe done
2020-10-15 14:11:02,374 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:02,375 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'pathloss', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:11:02,376 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2020-10-15 14:11:02,377 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss done
2020-10-15 14:11:03,153 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:03,155 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'barshadow', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:11:03,157 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2020-10-15 14:11:03,159 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow done
2020-10-15 14:11:03,891 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:03,894 - stpipe.Spec2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:11:03,908 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0057.fits
2020-10-15 14:11:03,909 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2020-10-15 14:11:04,829 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-15 14:11:04,830 - stpipe.Spec2Pipeline.photom - INFO - detector: MIRIFULONG
2020-10-15 14:11:04,830 - stpipe.Spec2Pipeline.photom - INFO - exp_type: MIR_MRS
2020-10-15 14:11:04,830 - stpipe.Spec2Pipeline.photom - INFO - band: SHORT
2020-10-15 14:11:04,895 - stpipe.Spec2Pipeline.photom - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:04,909 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2020-10-15 14:11:05,694 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure9_cal.fits>,).
2020-10-15 14:11:05,697 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'cube_build', 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'multi', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}
2020-10-15 14:11:05,698 - stpipe.Spec2Pipeline.cube_build - INFO - Step skipped.
2020-10-15 14:11:05,699 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build done
2020-10-15 14:11:06,432 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure9_cal.fits>,).
2020-10-15 14:11:06,434 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': True, 'suffix': 'x1d', 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}
2020-10-15 14:11:06,435 - stpipe.Spec2Pipeline.extract_1d - INFO - Step skipped.
2020-10-15 14:11:06,436 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2020-10-15 14:11:06,437 - stpipe.Spec2Pipeline - INFO - Finished processing product my_point_source_exposure9
2020-10-15 14:11:06,437 - stpipe.Spec2Pipeline - INFO - Processing product my_point_source_exposure10
2020-10-15 14:11:06,438 - stpipe.Spec2Pipeline - INFO - Working on input MRS_science/SHORT/det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits ...
2020-10-15 14:11:07,181 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:07,183 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-15 14:11:10,855 - stpipe.Spec2Pipeline.assign_wcs - INFO - Created a MIRI mir_mrs pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0031.asdf', 'filteroffset': None, 'specwcs': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0024.asdf', 'regions': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0027.asdf', 'wavelengthrange': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf', 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-15 14:11:11,874 - stpipe.Spec2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.000000000 -0.000681192 360.000000000 -0.000681192 360.000000000 0.000707138 0.000000000 0.000707138
2020-10-15 14:11:11,875 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-15 14:11:11,880 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-15 14:11:12,739 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits>, []).
2020-10-15 14:11:12,741 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'bkg_subtract', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-15 14:11:12,742 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step skipped.
2020-10-15 14:11:12,743 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-15 14:11:13,499 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits>, []).
2020-10-15 14:11:13,500 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'imprint_subtract', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:11:13,501 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2020-10-15 14:11:13,502 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract done
2020-10-15 14:11:14,252 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:14,254 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'msa_flagging', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:11:14,255 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2020-10-15 14:11:14,256 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging done
2020-10-15 14:11:15,014 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:15,016 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'srctype', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:11:15,025 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is MIR_MRS
2020-10-15 14:11:15,026 - stpipe.Spec2Pipeline.srctype - INFO - Input SRCTYAPT = None
2020-10-15 14:11:15,026 - stpipe.Spec2Pipeline.srctype - WARNING - SRCTYAPT keyword not found in input; using SRCTYPE instead
2020-10-15 14:11:15,027 - stpipe.Spec2Pipeline.srctype - INFO - Input source type is unknown; setting default SRCTYPE = EXTENDED
2020-10-15 14:11:15,028 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2020-10-15 14:11:15,921 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:15,923 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-15 14:11:16,018 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:16,019 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:16,020 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:16,026 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword DIFF_PATTERN does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:17,424 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2020-10-15 14:11:18,163 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:18,165 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'straylight', 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}
2020-10-15 14:11:18,176 - stpipe.Spec2Pipeline.straylight - INFO - Using regions reference file /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0027.asdf
2020-10-15 14:11:18,201 - stpipe.Spec2Pipeline.straylight - INFO - Using 20% throughput threshhold.
2020-10-15 14:11:18,202 - stpipe.Spec2Pipeline.straylight - INFO - Modified Shepard weighting power 1.00
2020-10-15 14:11:18,202 - stpipe.Spec2Pipeline.straylight - INFO - Region of influence radius (pixels) 50.00
2020-10-15 14:11:24,154 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight done
2020-10-15 14:11:24,957 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:24,959 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'fringe', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:11:24,970 - stpipe.Spec2Pipeline.fringe - INFO - Using FRINGE reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0046.fits
2020-10-15 14:11:25,013 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:25,014 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:25,014 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:26,302 - stpipe.Spec2Pipeline.fringe - INFO - DQ values in the reference file NOT used to update the output DQ.
2020-10-15 14:11:26,304 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe done
2020-10-15 14:11:27,123 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:27,124 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'pathloss', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:11:27,125 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2020-10-15 14:11:27,126 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss done
2020-10-15 14:11:27,854 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:27,856 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'barshadow', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:11:27,857 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2020-10-15 14:11:27,857 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow done
2020-10-15 14:11:28,591 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<IFUImageModel(1024, 1032) from det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:28,592 - stpipe.Spec2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:11:28,605 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0052.fits
2020-10-15 14:11:28,605 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2020-10-15 14:11:29,862 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-15 14:11:29,863 - stpipe.Spec2Pipeline.photom - INFO - detector: MIRIFUSHORT
2020-10-15 14:11:29,863 - stpipe.Spec2Pipeline.photom - INFO - exp_type: MIR_MRS
2020-10-15 14:11:29,863 - stpipe.Spec2Pipeline.photom - INFO - band: SHORT
2020-10-15 14:11:29,925 - stpipe.Spec2Pipeline.photom - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:29,939 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2020-10-15 14:11:30,843 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure10_cal.fits>,).
2020-10-15 14:11:30,846 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'cube_build', 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'multi', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}
2020-10-15 14:11:30,847 - stpipe.Spec2Pipeline.cube_build - INFO - Step skipped.
2020-10-15 14:11:30,848 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build done
2020-10-15 14:11:31,716 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure10_cal.fits>,).
2020-10-15 14:11:31,718 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': True, 'suffix': 'x1d', 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}
2020-10-15 14:11:31,719 - stpipe.Spec2Pipeline.extract_1d - INFO - Step skipped.
2020-10-15 14:11:31,721 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2020-10-15 14:11:31,722 - stpipe.Spec2Pipeline - INFO - Finished processing product my_point_source_exposure10
2020-10-15 14:11:31,722 - stpipe.Spec2Pipeline - INFO - Processing product my_point_source_exposure11
2020-10-15 14:11:31,723 - stpipe.Spec2Pipeline - INFO - Working on input MRS_science/SHORT/det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits ...
2020-10-15 14:11:32,563 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:32,564 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-15 14:11:35,524 - stpipe.Spec2Pipeline.assign_wcs - INFO - Created a MIRI mir_mrs pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0034.asdf', 'filteroffset': None, 'specwcs': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0020.asdf', 'regions': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0029.asdf', 'wavelengthrange': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf', 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-15 14:11:36,311 - stpipe.Spec2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.000000000 -0.001395265 359.999999996 -0.001395265 359.999999996 0.000866800 0.000000000 0.000866800
2020-10-15 14:11:36,311 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-15 14:11:36,316 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-15 14:11:37,119 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits>, []).
2020-10-15 14:11:37,121 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'bkg_subtract', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-15 14:11:37,122 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step skipped.
2020-10-15 14:11:37,122 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-15 14:11:37,845 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits>, []).
2020-10-15 14:11:37,846 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'imprint_subtract', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:11:37,847 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2020-10-15 14:11:37,848 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract done
2020-10-15 14:11:38,564 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:38,566 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'msa_flagging', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:11:38,567 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2020-10-15 14:11:38,568 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging done
2020-10-15 14:11:39,287 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:39,288 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'srctype', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:11:39,298 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is MIR_MRS
2020-10-15 14:11:39,299 - stpipe.Spec2Pipeline.srctype - INFO - Input SRCTYAPT = None
2020-10-15 14:11:39,301 - stpipe.Spec2Pipeline.srctype - WARNING - SRCTYAPT keyword not found in input; using SRCTYPE instead
2020-10-15 14:11:39,302 - stpipe.Spec2Pipeline.srctype - INFO - Input source type is unknown; setting default SRCTYPE = EXTENDED
2020-10-15 14:11:39,304 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2020-10-15 14:11:40,032 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:40,034 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-15 14:11:40,099 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:41,053 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2020-10-15 14:11:41,778 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:41,780 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'straylight', 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}
2020-10-15 14:11:41,788 - stpipe.Spec2Pipeline.straylight - WARNING - Straylight correction not defined for detector MIRIFULONG
2020-10-15 14:11:41,789 - stpipe.Spec2Pipeline.straylight - WARNING - Straylight step will be skipped
2020-10-15 14:11:42,698 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight done
2020-10-15 14:11:43,532 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:43,534 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'fringe', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:11:43,545 - stpipe.Spec2Pipeline.fringe - INFO - Using FRINGE reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0042.fits
2020-10-15 14:11:43,588 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:43,589 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:43,590 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:44,561 - stpipe.Spec2Pipeline.fringe - INFO - DQ values in the reference file NOT used to update the output DQ.
2020-10-15 14:11:44,564 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe done
2020-10-15 14:11:45,456 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:45,457 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'pathloss', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:11:45,458 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2020-10-15 14:11:45,459 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss done
2020-10-15 14:11:46,282 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:46,284 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'barshadow', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:11:46,285 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2020-10-15 14:11:46,286 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow done
2020-10-15 14:11:47,073 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits>,).
2020-10-15 14:11:47,075 - stpipe.Spec2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:11:47,095 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0057.fits
2020-10-15 14:11:47,097 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2020-10-15 14:11:48,141 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-15 14:11:48,142 - stpipe.Spec2Pipeline.photom - INFO - detector: MIRIFULONG
2020-10-15 14:11:48,143 - stpipe.Spec2Pipeline.photom - INFO - exp_type: MIR_MRS
2020-10-15 14:11:48,144 - stpipe.Spec2Pipeline.photom - INFO - band: SHORT
2020-10-15 14:11:48,211 - stpipe.Spec2Pipeline.photom - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:48,227 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2020-10-15 14:11:49,317 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure11_cal.fits>,).
2020-10-15 14:11:49,320 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'cube_build', 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'multi', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}
2020-10-15 14:11:49,323 - stpipe.Spec2Pipeline.cube_build - INFO - Step skipped.
2020-10-15 14:11:49,324 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build done
2020-10-15 14:11:50,168 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure11_cal.fits>,).
2020-10-15 14:11:50,170 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': True, 'suffix': 'x1d', 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}
2020-10-15 14:11:50,171 - stpipe.Spec2Pipeline.extract_1d - INFO - Step skipped.
2020-10-15 14:11:50,172 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2020-10-15 14:11:50,172 - stpipe.Spec2Pipeline - INFO - Finished processing product my_point_source_exposure11
2020-10-15 14:11:50,173 - stpipe.Spec2Pipeline - INFO - Processing product my_point_source_exposure12
2020-10-15 14:11:50,173 - stpipe.Spec2Pipeline - INFO - Working on input MRS_science/SHORT/det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits ...
2020-10-15 14:11:51,024 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:51,026 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-15 14:11:54,562 - stpipe.Spec2Pipeline.assign_wcs - INFO - Created a MIRI mir_mrs pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0031.asdf', 'filteroffset': None, 'specwcs': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_specwcs_0024.asdf', 'regions': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0027.asdf', 'wavelengthrange': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_wavelengthrange_0004.asdf', 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-15 14:11:55,446 - stpipe.Spec2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.000000001 -0.000870707 360.000000000 -0.000870707 360.000000000 0.000517623 0.000000001 0.000517623
2020-10-15 14:11:55,447 - stpipe.Spec2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-15 14:11:55,451 - stpipe.Spec2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-15 14:11:56,299 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits>, []).
2020-10-15 14:11:56,300 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'bkg_subtract', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-15 14:11:56,301 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step skipped.
2020-10-15 14:11:56,302 - stpipe.Spec2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-15 14:11:57,066 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits>, []).
2020-10-15 14:11:57,068 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'imprint_subtract', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:11:57,068 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step skipped.
2020-10-15 14:11:57,069 - stpipe.Spec2Pipeline.imprint_subtract - INFO - Step imprint_subtract done
2020-10-15 14:11:57,821 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:57,822 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'msa_flagging', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:11:57,823 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step skipped.
2020-10-15 14:11:57,824 - stpipe.Spec2Pipeline.msa_flagging - INFO - Step msa_flagging done
2020-10-15 14:11:58,659 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:58,660 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'srctype', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:11:58,670 - stpipe.Spec2Pipeline.srctype - INFO - Input EXP_TYPE is MIR_MRS
2020-10-15 14:11:58,670 - stpipe.Spec2Pipeline.srctype - INFO - Input SRCTYAPT = None
2020-10-15 14:11:58,671 - stpipe.Spec2Pipeline.srctype - WARNING - SRCTYAPT keyword not found in input; using SRCTYPE instead
2020-10-15 14:11:58,672 - stpipe.Spec2Pipeline.srctype - INFO - Input source type is unknown; setting default SRCTYPE = EXTENDED
2020-10-15 14:11:58,673 - stpipe.Spec2Pipeline.srctype - INFO - Step srctype done
2020-10-15 14:11:59,447 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:11:59,448 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-15 14:11:59,511 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:59,512 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:59,512 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:11:59,517 - stpipe.Spec2Pipeline.flat_field - WARNING - Keyword DIFF_PATTERN does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:12:00,811 - stpipe.Spec2Pipeline.flat_field - INFO - Step flat_field done
2020-10-15 14:12:01,578 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:12:01,580 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'straylight', 'search_output_file': True, 'input_dir': '', 'method': 'ModShepard', 'roi': 50, 'power': 1.0}
2020-10-15 14:12:01,590 - stpipe.Spec2Pipeline.straylight - INFO - Using regions reference file /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_regions_0027.asdf
2020-10-15 14:12:01,615 - stpipe.Spec2Pipeline.straylight - INFO - Using 20% throughput threshhold.
2020-10-15 14:12:01,616 - stpipe.Spec2Pipeline.straylight - INFO - Modified Shepard weighting power 1.00
2020-10-15 14:12:01,616 - stpipe.Spec2Pipeline.straylight - INFO - Region of influence radius (pixels) 50.00
2020-10-15 14:12:07,693 - stpipe.Spec2Pipeline.straylight - INFO - Step straylight done
2020-10-15 14:12:08,619 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:12:08,621 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'fringe', 'search_output_file': True, 'input_dir': ''}
2020-10-15 14:12:08,631 - stpipe.Spec2Pipeline.fringe - INFO - Using FRINGE reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_fringe_0046.fits
2020-10-15 14:12:08,673 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:12:08,674 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:12:08,675 - stpipe.Spec2Pipeline.fringe - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:12:09,912 - stpipe.Spec2Pipeline.fringe - INFO - DQ values in the reference file NOT used to update the output DQ.
2020-10-15 14:12:09,914 - stpipe.Spec2Pipeline.fringe - INFO - Step fringe done
2020-10-15 14:12:10,779 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:12:10,781 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'pathloss', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:12:10,781 - stpipe.Spec2Pipeline.pathloss - INFO - Step skipped.
2020-10-15 14:12:10,782 - stpipe.Spec2Pipeline.pathloss - INFO - Step pathloss done
2020-10-15 14:12:11,579 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:12:11,581 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'barshadow', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:12:11,582 - stpipe.Spec2Pipeline.barshadow - INFO - Step skipped.
2020-10-15 14:12:11,583 - stpipe.Spec2Pipeline.barshadow - INFO - Step barshadow done
2020-10-15 14:12:12,462 - stpipe.Spec2Pipeline.photom - INFO - Step photom running with args (<IFUImageModel(1024, 1032) from det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits>,).
2020-10-15 14:12:12,464 - stpipe.Spec2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-15 14:12:12,476 - stpipe.Spec2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0052.fits
2020-10-15 14:12:12,477 - stpipe.Spec2Pipeline.photom - INFO - Using area reference file: N/A
2020-10-15 14:12:13,722 - stpipe.Spec2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-15 14:12:13,723 - stpipe.Spec2Pipeline.photom - INFO - detector: MIRIFUSHORT
2020-10-15 14:12:13,723 - stpipe.Spec2Pipeline.photom - INFO - exp_type: MIR_MRS
2020-10-15 14:12:13,724 - stpipe.Spec2Pipeline.photom - INFO - band: SHORT
2020-10-15 14:12:13,783 - stpipe.Spec2Pipeline.photom - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-15 14:12:13,796 - stpipe.Spec2Pipeline.photom - INFO - Step photom done
2020-10-15 14:12:14,704 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure12_cal.fits>,).
2020-10-15 14:12:14,706 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': True, 'output_use_index': True, 'save_results': False, 'skip': True, 'suffix': 'cube_build', 'search_output_file': False, 'input_dir': '', 'channel': 'all', 'band': 'all', 'grating': 'all', 'filter': 'all', 'output_type': 'multi', 'scale1': 0.0, 'scale2': 0.0, 'scalew': 0.0, 'weighting': 'emsm', 'coord_system': 'skyalign', 'rois': 0.0, 'roiw': 0.0, 'weight_power': 0.0, 'wavemin': None, 'wavemax': None, 'single': False, 'xdebug': None, 'ydebug': None, 'zdebug': None, 'skip_dqflagging': False}
2020-10-15 14:12:14,707 - stpipe.Spec2Pipeline.cube_build - INFO - Step skipped.
2020-10-15 14:12:14,708 - stpipe.Spec2Pipeline.cube_build - INFO - Step cube_build done
2020-10-15 14:12:15,543 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d running with args (<IFUImageModel(1024, 1032) from MRS_science/my_point_source_exposure12_cal.fits>,).
2020-10-15 14:12:15,545 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': True, 'suffix': 'x1d', 'search_output_file': True, 'input_dir': '', 'smoothing_length': None, 'bkg_order': None, 'log_increment': 50, 'subtract_background': None, 'use_source_posn': None, 'apply_apcorr': True}
2020-10-15 14:12:15,546 - stpipe.Spec2Pipeline.extract_1d - INFO - Step skipped.
2020-10-15 14:12:15,547 - stpipe.Spec2Pipeline.extract_1d - INFO - Step extract_1d done
2020-10-15 14:12:15,547 - stpipe.Spec2Pipeline - INFO - Finished processing product my_point_source_exposure12
2020-10-15 14:12:15,548 - stpipe.Spec2Pipeline - INFO - Ending calwebb_spec2
2020-10-15 14:12:16,487 - stpipe.Spec2Pipeline - INFO - Saved model in MRS_science/my_point_source_exposure1_cal.fits
2020-10-15 14:12:17,610 - stpipe.Spec2Pipeline - INFO - Saved model in MRS_science/my_point_source_exposure2_cal.fits
2020-10-15 14:12:18,517 - stpipe.Spec2Pipeline - INFO - Saved model in MRS_science/my_point_source_exposure3_cal.fits
2020-10-15 14:12:19,638 - stpipe.Spec2Pipeline - INFO - Saved model in MRS_science/my_point_source_exposure4_cal.fits
2020-10-15 14:12:21,430 - stpipe.Spec2Pipeline - INFO - Saved model in MRS_science/my_point_source_exposure5_cal.fits
2020-10-15 14:12:22,556 - stpipe.Spec2Pipeline - INFO - Saved model in MRS_science/my_point_source_exposure6_cal.fits
2020-10-15 14:12:23,580 - stpipe.Spec2Pipeline - INFO - Saved model in MRS_science/my_point_source_exposure7_cal.fits
2020-10-15 14:12:25,004 - stpipe.Spec2Pipeline - INFO - Saved model in MRS_science/my_point_source_exposure8_cal.fits
2020-10-15 14:12:26,971 - stpipe.Spec2Pipeline - INFO - Saved model in MRS_science/my_point_source_exposure9_cal.fits
2020-10-15 14:12:28,197 - stpipe.Spec2Pipeline - INFO - Saved model in MRS_science/my_point_source_exposure10_cal.fits
2020-10-15 14:12:29,186 - stpipe.Spec2Pipeline - INFO - Saved model in MRS_science/my_point_source_exposure11_cal.fits
2020-10-15 14:12:30,421 - stpipe.Spec2Pipeline - INFO - Saved model in MRS_science/my_point_source_exposure12_cal.fits
2020-10-15 14:12:30,422 - stpipe.Spec2Pipeline - INFO - Step Spec2Pipeline done
[<IFUImageModel(1024, 1032) from my_point_source_exposure1_cal.fits>,
<IFUImageModel(1024, 1032) from my_point_source_exposure2_cal.fits>,
<IFUImageModel(1024, 1032) from my_point_source_exposure3_cal.fits>,
<IFUImageModel(1024, 1032) from my_point_source_exposure4_cal.fits>,
<IFUImageModel(1024, 1032) from my_point_source_exposure5_cal.fits>,
<IFUImageModel(1024, 1032) from my_point_source_exposure6_cal.fits>,
<IFUImageModel(1024, 1032) from my_point_source_exposure7_cal.fits>,
<IFUImageModel(1024, 1032) from my_point_source_exposure8_cal.fits>,
<IFUImageModel(1024, 1032) from my_point_source_exposure9_cal.fits>,
<IFUImageModel(1024, 1032) from my_point_source_exposure10_cal.fits>,
<IFUImageModel(1024, 1032) from my_point_source_exposure11_cal.fits>,
<IFUImageModel(1024, 1032) from my_point_source_exposure12_cal.fits>]
We can plot the before (rate) and after (cal) images for the first dither position of the SHORT band
# use the association to identify the linked files
cal_file = asn['products'][9]['name'] + '.fits'
rate_file = asn['products'][9]['members'][0]['expname']
# open the input level 2A image and calibrated 2B image a jwst data models
with datamodels.open(rate_file) as in_dm:
with datamodels.open(rate_file) as out_dm:
fig, axs = plt.subplots(1, 2, figsize=(14, 7), sharey=True)
axs[0].imshow(in_dm.data, cmap='jet', interpolation='nearest', origin='lower', norm=LogNorm(vmin=1, vmax=100))
axs[0].annotate('DMS Level 2A: {}'.format(os.path.basename(rate_file)), xy=(0.0, 1.02), xycoords='axes fraction',
fontsize=10, fontweight='bold', color='k')
axs[0].set_facecolor('black')
axs[1].imshow(out_dm.data, cmap='jet', interpolation='nearest', origin='lower', norm=LogNorm(vmin=1, vmax=100))
axs[1].annotate('DMS Level 2B: {}'.format(cal_file), xy=(0.0, 1.02), xycoords='axes fraction',
fontsize=10, fontweight='bold', color='k')
axs[1].set_facecolor('black')
plt.tight_layout()
plt.show()
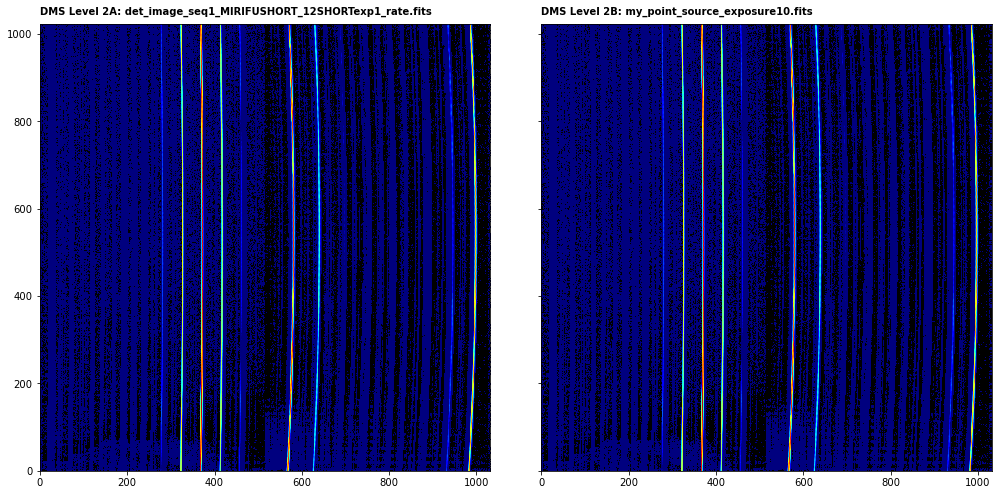
Command line¶
To achieve the same result from the command line there are a couple of options. However, one must still generate the association file. In this case, it is best to copy the template above into a text file and save it to my_point_source_lvl2_asn.json
. The content is printed here for convenience.
{
"asn_type": "spec2",
"asn_rule": "DMSLevel2bBase",
"version_id": null,
"code_version": "0.13.7",
"degraded_status": "No known degraded exposures in association.",
"program": "noprogram",
"products": [
{
"name": "my_point_source_exposure1",
"members": [
{
"expname": "MRS/LONG/det_image_seq1_MIRIFULONG_34LONGexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure2",
"members": [
{
"expname": "MRS/LONG/det_image_seq1_MIRIFUSHORT_12LONGexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure3",
"members": [
{
"expname": "MRS/LONG/det_image_seq2_MIRIFULONG_34LONGexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure4",
"members": [
{
"expname": "MRS/LONG/det_image_seq2_MIRIFUSHORT_12LONGexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure5",
"members": [
{
"expname": "MRS/MEDIUM/det_image_seq1_MIRIFULONG_34MEDIUMexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure6",
"members": [
{
"expname": "MRS/MEDIUM/det_image_seq1_MIRIFUSHORT_12MEDIUMexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure7",
"members": [
{
"expname": "MRS/MEDIUM/det_image_seq2_MIRIFULONG_34MEDIUMexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure8",
"members": [
{
"expname": "MRS/MEDIUM/det_image_seq2_MIRIFUSHORT_12MEDIUMexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure9",
"members": [
{
"expname": "MRS/SHORT/det_image_seq1_MIRIFULONG_34SHORTexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure10",
"members": [
{
"expname": "MRS/SHORT/det_image_seq1_MIRIFUSHORT_12SHORTexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure11",
"members": [
{
"expname": "MRS/SHORT/det_image_seq2_MIRIFULONG_34SHORTexp1_rate.fits",
"exptype": "science"
}
]
},
{
"name": "my_point_source_exposure12",
"members": [
{
"expname": "MRS/SHORT/det_image_seq2_MIRIFUSHORT_12SHORTexp1_rate.fits",
"exptype": "science"
}
]
}
],
"asn_pool": "my_point_source_pool"
}
Option 1:
Run the Spec2Pipeline
class using the strun
command:
mkdir demo_output
strun jwst.pipeline.Spec2Pipeline my_point_source_lvl2_asn.json --output_dir demo_output --steps.photom.save_results True --steps.cube_build.skip True --steps.extract_1d.skip True
This will produce the same output file in the user-defined --output_dir
Option 2:
Collect the pipeline configuration files in your working directory (if they are not already there) using collect_pipeline_configs
and then run the Spec2Pipeline
using the strun
command with the associated calwebb_spec2.cfg
file. This option is a little more flexible as one can create edit the cfg files, use them again, etc.
mkdir demo_output
collect_pipeline_cfgs cfgs/
strun cfgs/calwebb_spec2.cfg my_point_source_lvl2_asn.json --output_dir demo_output --steps.photom.save_results True --steps.cube_build.skip True --steps.extract_1d.skip True
This will produce the same output file in the user-defined --output_dir