Image2Pipeline (MIRI CALIMAGE2)¶
Image2Pipeline
applies instrumental corrections and calibrations, in addition to the subtraction of dedicated background exposures, that result in a fully calibrated, background subtracted individual exposures.
Official documentation for Image2Pipeline
can be found here:
https://jwst-pipeline.readthedocs.io/en/latest/jwst/pipeline/calwebb_image2.html
The Image2Pipeline
comprises a linear series of steps. The steps applied to MIRI data in order are:
Step |
Description |
---|---|
|
subtract dedicated background exposures |
|
attach WCS object to each science exposure |
|
correct the exposure using the flat-field reference file |
|
apply flux (photometric) calibration |
|
resample using WCS and distortion information into an undistorted product |
Input data¶
An example of running the file through the Image2Pipeline
is now shown using a simple simulated 4-point dither observation of a galaxy with the MIRI Imager (F1130W filter) produced with MIRISim v2.3 and brought to level 2A using the Detector1Pipeline
. A background observation is also associated to the science exposures for subtraction.
Python¶
Start by importing what will be used and set the CRDS_CONTEXT
# imports
import os, glob, shutil
import numpy as np
from matplotlib.colors import LogNorm
import matplotlib.pyplot as plt
from subprocess import call
from jwst import datamodels
from jwst.associations.lib.member import Member
from jwst.associations.asn_from_list import asn_from_list
from jwst.associations.lib.rules_level2_base import DMSLevel2bBase
# set the CRDS_CONTEXT
os.environ["CRDS_CONTEXT"] = "jwst_0641.pmap"
Image2Pipeline
can be run by passing indivual exposures or datamodels but in this sample case we produce an association file that links the background exposures to the science exposures so it can be subtracted. It is assumed that the science files are in ‘IMA_science’ and the background file is in ‘IMA_background’. The content of the association file is printed for inspection.
# load the science and background files to lists
my_science_files = glob.glob('IMA_science/*rate.fits')
my_background_files = glob.glob('IMA_background/*rate.fits')
my_science_files = sorted(my_science_files)
# setup an empty level 2 association structure
asn = asn_from_list(my_science_files, rule=DMSLevel2bBase)
asn.data['products'] = None
# set the association name
asn_name = 'my_galaxy'
# set some metadata
asn['asn_pool'] = asn_name + '_pool'
asn['asn_type'] = 'image2'
for n, sci in enumerate(my_science_files):
asn.new_product('{}_dither{}'.format(asn_name, str(n+1)))
sci_member = Member({'expname': sci, 'exptype': 'science'})
new_members = asn.current_product['members']
new_members.append(sci_member)
for bkg in my_background_files:
bkg_member = Member({'expname': bkg, 'exptype': 'background'})
new_members.append(bkg_member)
# print the association and save to file
name, ser = asn.dump()
print(ser)
asn_file = asn_name + '_lvl2_asn.json'
with open(asn_file, 'w') as f:
f.write(ser)
{
"asn_type": "image2",
"asn_rule": "DMSLevel2bBase",
"version_id": null,
"code_version": "0.17.1",
"degraded_status": "No known degraded exposures in association.",
"program": "noprogram",
"constraints": "No constraints",
"asn_id": "a3001",
"asn_pool": "my_galaxy_pool",
"products": [
{
"name": "my_galaxy_dither1",
"members": [
{
"expname": "IMA_science/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "science"
},
{
"expname": "IMA_background/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "background"
}
]
},
{
"name": "my_galaxy_dither2",
"members": [
{
"expname": "IMA_science/det_image_seq2_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "science"
},
{
"expname": "IMA_background/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "background"
}
]
},
{
"name": "my_galaxy_dither3",
"members": [
{
"expname": "IMA_science/det_image_seq3_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "science"
},
{
"expname": "IMA_background/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "background"
}
]
},
{
"name": "my_galaxy_dither4",
"members": [
{
"expname": "IMA_science/det_image_seq4_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "science"
},
{
"expname": "IMA_background/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "background"
}
]
}
]
}
Import Image2Pipeline and print the docstring to show some information
from jwst.pipeline import Image2Pipeline
print(Image2Pipeline.__doc__)
Image2Pipeline: Processes JWST imaging-mode slope data from Level-2a to
Level-2b.
Included steps are:
background_subtraction, assign_wcs, flat_field, photom and resample.
The association file is passed as input. The output level 2B files will be saved in my_output_dir
as _cal.fits
.
Parameters used:
save_results
: boolean, optional, default=False
save the results to file
output_dir
: boolean, optional, default is the working directory
the location to save the output
Note that the Image2Pipeline
will return the level 2B datamodel for each exposure as a list of datamodels so we set these to dm_cont
. The output filenames will be determined by the asn_name
in the association file which was set in the cell above.
# user specified
my_output_dir = 'demo_output'
# the output directory should be created if it doesn't exist
if not os.path.exists(my_output_dir):
os.mkdir(my_output_dir)
# run the pipeline
dm_cont = Image2Pipeline.call(asn_file, save_results=True, output_dir=my_output_dir)
2020-10-14 14:22:35,344 - CRDS - ERROR - Error determining best reference for 'pars-backgroundstep' = Unknown reference type 'pars-backgroundstep'
2020-10-14 14:22:35,443 - CRDS - ERROR - Error determining best reference for 'pars-assignwcsstep' = Unknown reference type 'pars-assignwcsstep'
2020-10-14 14:22:35,489 - CRDS - ERROR - Error determining best reference for 'pars-flatfieldstep' = Unknown reference type 'pars-flatfieldstep'
2020-10-14 14:22:35,535 - CRDS - ERROR - Error determining best reference for 'pars-photomstep' = Unknown reference type 'pars-photomstep'
2020-10-14 14:22:35,583 - CRDS - ERROR - Error determining best reference for 'pars-resamplestep' = Unknown reference type 'pars-resamplestep'
2020-10-14 14:22:35,628 - CRDS - ERROR - Error determining best reference for 'pars-image2pipeline' = Unknown reference type 'pars-image2pipeline'
2020-10-14 14:22:35,633 - stpipe.Image2Pipeline - INFO - Image2Pipeline instance created.
2020-10-14 14:22:35,635 - stpipe.Image2Pipeline.bkg_subtract - INFO - BackgroundStep instance created.
2020-10-14 14:22:35,636 - stpipe.Image2Pipeline.assign_wcs - INFO - AssignWcsStep instance created.
2020-10-14 14:22:35,638 - stpipe.Image2Pipeline.flat_field - INFO - FlatFieldStep instance created.
2020-10-14 14:22:35,640 - stpipe.Image2Pipeline.photom - INFO - PhotomStep instance created.
2020-10-14 14:22:35,642 - stpipe.Image2Pipeline.resample - INFO - ResampleStep instance created.
2020-10-14 14:22:35,721 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline running with args ('my_galaxy_lvl2_asn.json',).
2020-10-14 14:22:35,726 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': 'demo_output', 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'save_bsub': False, 'steps': {'bkg_subtract': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}, 'assign_wcs': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}, 'flat_field': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}, 'photom': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}, 'resample': {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'pixfrac': 1.0, 'kernel': 'square', 'fillval': 'INDEF', 'weight_type': 'exptime', 'single': False, 'blendheaders': True}}}
2020-10-14 14:22:35,793 - stpipe.Image2Pipeline - INFO - Prefetching reference files for dataset: 'det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits' reftypes = ['area', 'camera', 'collimator', 'dflat', 'disperser', 'distortion', 'drizpars', 'fflat', 'filteroffset', 'flat', 'fore', 'fpa', 'ifufore', 'ifupost', 'ifuslicer', 'msa', 'ote', 'photom', 'regions', 'sflat', 'specwcs', 'wavelengthrange', 'wfssbkg']
2020-10-14 14:22:35,801 - stpipe.Image2Pipeline - INFO - Prefetch for AREA reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_area_0004.fits'.
2020-10-14 14:22:35,802 - stpipe.Image2Pipeline - INFO - Prefetch for CAMERA reference file is 'N/A'.
2020-10-14 14:22:35,802 - stpipe.Image2Pipeline - INFO - Prefetch for COLLIMATOR reference file is 'N/A'.
2020-10-14 14:22:35,803 - stpipe.Image2Pipeline - INFO - Prefetch for DFLAT reference file is 'N/A'.
2020-10-14 14:22:35,803 - stpipe.Image2Pipeline - INFO - Prefetch for DISPERSER reference file is 'N/A'.
2020-10-14 14:22:35,804 - stpipe.Image2Pipeline - INFO - Prefetch for DISTORTION reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0028.asdf'.
2020-10-14 14:22:35,805 - stpipe.Image2Pipeline - INFO - Prefetch for DRIZPARS reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_drizpars_0001.fits'.
2020-10-14 14:22:35,806 - stpipe.Image2Pipeline - INFO - Prefetch for FFLAT reference file is 'N/A'.
2020-10-14 14:22:35,807 - stpipe.Image2Pipeline - INFO - Prefetch for FILTEROFFSET reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_filteroffset_0005.asdf'.
2020-10-14 14:22:35,808 - stpipe.Image2Pipeline - INFO - Prefetch for FLAT reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_flat_0508.fits'.
2020-10-14 14:22:35,809 - stpipe.Image2Pipeline - INFO - Prefetch for FORE reference file is 'N/A'.
2020-10-14 14:22:35,809 - stpipe.Image2Pipeline - INFO - Prefetch for FPA reference file is 'N/A'.
2020-10-14 14:22:35,810 - stpipe.Image2Pipeline - INFO - Prefetch for IFUFORE reference file is 'N/A'.
2020-10-14 14:22:35,810 - stpipe.Image2Pipeline - INFO - Prefetch for IFUPOST reference file is 'N/A'.
2020-10-14 14:22:35,811 - stpipe.Image2Pipeline - INFO - Prefetch for IFUSLICER reference file is 'N/A'.
2020-10-14 14:22:35,812 - stpipe.Image2Pipeline - INFO - Prefetch for MSA reference file is 'N/A'.
2020-10-14 14:22:35,812 - stpipe.Image2Pipeline - INFO - Prefetch for OTE reference file is 'N/A'.
2020-10-14 14:22:35,813 - stpipe.Image2Pipeline - INFO - Prefetch for PHOTOM reference file is '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0044.fits'.
2020-10-14 14:22:35,814 - stpipe.Image2Pipeline - INFO - Prefetch for REGIONS reference file is 'N/A'.
2020-10-14 14:22:35,815 - stpipe.Image2Pipeline - INFO - Prefetch for SFLAT reference file is 'N/A'.
2020-10-14 14:22:35,816 - stpipe.Image2Pipeline - INFO - Prefetch for SPECWCS reference file is 'N/A'.
2020-10-14 14:22:35,817 - stpipe.Image2Pipeline - INFO - Prefetch for WAVELENGTHRANGE reference file is 'N/A'.
2020-10-14 14:22:35,817 - stpipe.Image2Pipeline - INFO - Prefetch for WFSSBKG reference file is 'N/A'.
2020-10-14 14:22:35,818 - stpipe.Image2Pipeline - INFO - Starting calwebb_image2 ...
2020-10-14 14:22:35,830 - stpipe.Image2Pipeline - INFO - Processing product my_galaxy_dither1
2020-10-14 14:22:35,831 - stpipe.Image2Pipeline - INFO - Working on input IMA_science/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits ...
2020-10-14 14:22:35,930 - stpipe.Image2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(1024, 1032) from det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits>, ['IMA_background/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits']).
2020-10-14 14:22:35,932 - stpipe.Image2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'bsub', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-14 14:22:36,319 - stpipe.Image2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-14 14:22:36,372 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(1024, 1032) from det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:36,373 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-14 14:22:36,639 - stpipe.Image2Pipeline.assign_wcs - INFO - Created a MIRI mir_image pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0028.asdf', 'filteroffset': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_filteroffset_0005.asdf', 'specwcs': None, 'regions': None, 'wavelengthrange': None, 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-14 14:22:36,673 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.019410529 -0.016138544 0.021878136 0.015048625 359.990819026 0.017621279 359.988011958 -0.013579591
2020-10-14 14:22:36,674 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 0.019410529 -0.016138544 0.021878136 0.015048625 359.990819026 0.017621279 359.988011958 -0.013579591
2020-10-14 14:22:36,675 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-14 14:22:36,679 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-14 14:22:36,743 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(1024, 1032) from det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:36,745 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-14 14:22:36,835 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:36,836 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:36,836 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:36,843 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword DIFF_PATTERN does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:36,985 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2020-10-14 14:22:37,038 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(1024, 1032) from det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:37,039 - stpipe.Image2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': None, 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-14 14:22:37,050 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0044.fits
2020-10-14 14:22:37,050 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_area_0004.fits
2020-10-14 14:22:37,117 - stpipe.Image2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-14 14:22:37,117 - stpipe.Image2Pipeline.photom - INFO - detector: MIRIMAGE
2020-10-14 14:22:37,118 - stpipe.Image2Pipeline.photom - INFO - exp_type: MIR_IMAGE
2020-10-14 14:22:37,119 - stpipe.Image2Pipeline.photom - INFO - filter: F1130W
2020-10-14 14:22:37,171 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2020-10-14 14:22:37,172 - stpipe.Image2Pipeline.photom - INFO - subarray: FULL
2020-10-14 14:22:37,173 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 2.44947
2020-10-14 14:22:37,182 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2020-10-14 14:22:37,249 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(1024, 1032) from det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:37,250 - stpipe.Image2Pipeline.resample - INFO - Step resample parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': False, 'suffix': 'i2d', 'search_output_file': True, 'input_dir': '', 'pixfrac': 1.0, 'kernel': 'square', 'fillval': 'INDEF', 'weight_type': 'exptime', 'single': False, 'blendheaders': True}
2020-10-14 14:22:37,268 - stpipe.Image2Pipeline.resample - INFO - Drizpars reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_drizpars_0001.fits
2020-10-14 14:22:37,876 - stpipe.Image2Pipeline.resample - INFO - Drizzling (1024, 1032) --> (1023, 1023)
2020-10-14 14:22:38,308 - stpipe.Image2Pipeline.resample - INFO - Update S_REGION to POLYGON ICRS 0.019358646 -0.016334386 0.022108876 0.015005126 359.990738698 0.017758047 359.987988468 -0.013581465
2020-10-14 14:22:38,449 - stpipe.Image2Pipeline.resample - INFO - Saved model in demo_output/my_galaxy_dither1_i2d.fits
2020-10-14 14:22:38,450 - stpipe.Image2Pipeline.resample - INFO - Step resample done
2020-10-14 14:22:38,450 - stpipe.Image2Pipeline - INFO - Finished processing product my_galaxy_dither1
2020-10-14 14:22:38,451 - stpipe.Image2Pipeline - INFO - Processing product my_galaxy_dither2
2020-10-14 14:22:38,451 - stpipe.Image2Pipeline - INFO - Working on input IMA_science/det_image_seq2_MIRIMAGE_F1130Wexp1_rate.fits ...
2020-10-14 14:22:38,569 - stpipe.Image2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(1024, 1032) from det_image_seq2_MIRIMAGE_F1130Wexp1_rate.fits>, ['IMA_background/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits']).
2020-10-14 14:22:38,571 - stpipe.Image2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'bsub', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-14 14:22:38,935 - stpipe.Image2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-14 14:22:38,998 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(1024, 1032) from det_image_seq2_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:38,999 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-14 14:22:39,127 - stpipe.Image2Pipeline.assign_wcs - INFO - Created a MIRI mir_image pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0028.asdf', 'filteroffset': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_filteroffset_0005.asdf', 'specwcs': None, 'regions': None, 'wavelengthrange': None, 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-14 14:22:39,162 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.020049678 -0.017460337 0.022517284 0.013726832 359.991458175 0.016299486 359.988651107 -0.014901383
2020-10-14 14:22:39,163 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 0.020049678 -0.017460337 0.022517284 0.013726832 359.991458175 0.016299486 359.988651107 -0.014901383
2020-10-14 14:22:39,163 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-14 14:22:39,167 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-14 14:22:39,227 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(1024, 1032) from det_image_seq2_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:39,229 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-14 14:22:39,289 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:39,290 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:39,290 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:39,297 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword DIFF_PATTERN does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:39,389 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2020-10-14 14:22:39,456 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(1024, 1032) from det_image_seq2_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:39,458 - stpipe.Image2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-14 14:22:39,469 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0044.fits
2020-10-14 14:22:39,469 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_area_0004.fits
2020-10-14 14:22:39,536 - stpipe.Image2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-14 14:22:39,537 - stpipe.Image2Pipeline.photom - INFO - detector: MIRIMAGE
2020-10-14 14:22:39,537 - stpipe.Image2Pipeline.photom - INFO - exp_type: MIR_IMAGE
2020-10-14 14:22:39,538 - stpipe.Image2Pipeline.photom - INFO - filter: F1130W
2020-10-14 14:22:39,563 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2020-10-14 14:22:39,565 - stpipe.Image2Pipeline.photom - INFO - subarray: FULL
2020-10-14 14:22:39,565 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 2.44947
2020-10-14 14:22:39,573 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2020-10-14 14:22:39,635 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(1024, 1032) from det_image_seq2_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:39,636 - stpipe.Image2Pipeline.resample - INFO - Step resample parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': False, 'suffix': 'i2d', 'search_output_file': True, 'input_dir': '', 'pixfrac': 1.0, 'kernel': 'square', 'fillval': 'INDEF', 'weight_type': 'exptime', 'single': False, 'blendheaders': False}
2020-10-14 14:22:39,652 - stpipe.Image2Pipeline.resample - INFO - Drizpars reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_drizpars_0001.fits
2020-10-14 14:22:40,237 - stpipe.Image2Pipeline.resample - INFO - Drizzling (1024, 1032) --> (1023, 1023)
2020-10-14 14:22:40,671 - stpipe.Image2Pipeline.resample - INFO - Update S_REGION to POLYGON ICRS 0.019997794 -0.017656179 0.022748024 0.013683333 359.991377847 0.016436254 359.988627617 -0.014903258
2020-10-14 14:22:40,797 - stpipe.Image2Pipeline.resample - INFO - Saved model in demo_output/my_galaxy_dither2_i2d.fits
2020-10-14 14:22:40,798 - stpipe.Image2Pipeline.resample - INFO - Step resample done
2020-10-14 14:22:40,798 - stpipe.Image2Pipeline - INFO - Finished processing product my_galaxy_dither2
2020-10-14 14:22:40,799 - stpipe.Image2Pipeline - INFO - Processing product my_galaxy_dither3
2020-10-14 14:22:40,799 - stpipe.Image2Pipeline - INFO - Working on input IMA_science/det_image_seq3_MIRIMAGE_F1130Wexp1_rate.fits ...
2020-10-14 14:22:40,916 - stpipe.Image2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(1024, 1032) from det_image_seq3_MIRIMAGE_F1130Wexp1_rate.fits>, ['IMA_background/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits']).
2020-10-14 14:22:40,917 - stpipe.Image2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'bsub', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-14 14:22:41,291 - stpipe.Image2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-14 14:22:41,371 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(1024, 1032) from det_image_seq3_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:41,373 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-14 14:22:41,561 - stpipe.Image2Pipeline.assign_wcs - INFO - Created a MIRI mir_image pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0028.asdf', 'filteroffset': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_filteroffset_0005.asdf', 'specwcs': None, 'regions': None, 'wavelengthrange': None, 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-14 14:22:41,597 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.020280823 -0.019911110 0.022748429 0.011276059 359.991689320 0.013848713 359.988882252 -0.017352157
2020-10-14 14:22:41,598 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 0.020280823 -0.019911110 0.022748429 0.011276059 359.991689320 0.013848713 359.988882252 -0.017352157
2020-10-14 14:22:41,599 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-14 14:22:41,603 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-14 14:22:41,680 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(1024, 1032) from det_image_seq3_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:41,682 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-14 14:22:41,746 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:41,747 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:41,748 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:41,757 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword DIFF_PATTERN does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:41,861 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2020-10-14 14:22:41,936 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(1024, 1032) from det_image_seq3_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:41,938 - stpipe.Image2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-14 14:22:41,949 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0044.fits
2020-10-14 14:22:41,950 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_area_0004.fits
2020-10-14 14:22:42,021 - stpipe.Image2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-14 14:22:42,022 - stpipe.Image2Pipeline.photom - INFO - detector: MIRIMAGE
2020-10-14 14:22:42,022 - stpipe.Image2Pipeline.photom - INFO - exp_type: MIR_IMAGE
2020-10-14 14:22:42,023 - stpipe.Image2Pipeline.photom - INFO - filter: F1130W
2020-10-14 14:22:42,049 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2020-10-14 14:22:42,050 - stpipe.Image2Pipeline.photom - INFO - subarray: FULL
2020-10-14 14:22:42,051 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 2.44947
2020-10-14 14:22:42,060 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2020-10-14 14:22:42,129 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(1024, 1032) from det_image_seq3_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:42,131 - stpipe.Image2Pipeline.resample - INFO - Step resample parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': False, 'suffix': 'i2d', 'search_output_file': True, 'input_dir': '', 'pixfrac': 1.0, 'kernel': 'square', 'fillval': 'INDEF', 'weight_type': 'exptime', 'single': False, 'blendheaders': False}
2020-10-14 14:22:42,148 - stpipe.Image2Pipeline.resample - INFO - Drizpars reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_drizpars_0001.fits
2020-10-14 14:22:42,728 - stpipe.Image2Pipeline.resample - INFO - Drizzling (1024, 1032) --> (1023, 1023)
2020-10-14 14:22:43,150 - stpipe.Image2Pipeline.resample - INFO - Update S_REGION to POLYGON ICRS 0.020228939 -0.020106952 0.022979169 0.011232559 359.991608992 0.013985480 359.988858761 -0.017354031
2020-10-14 14:22:43,337 - stpipe.Image2Pipeline.resample - INFO - Saved model in demo_output/my_galaxy_dither3_i2d.fits
2020-10-14 14:22:43,338 - stpipe.Image2Pipeline.resample - INFO - Step resample done
2020-10-14 14:22:43,339 - stpipe.Image2Pipeline - INFO - Finished processing product my_galaxy_dither3
2020-10-14 14:22:43,340 - stpipe.Image2Pipeline - INFO - Processing product my_galaxy_dither4
2020-10-14 14:22:43,340 - stpipe.Image2Pipeline - INFO - Working on input IMA_science/det_image_seq4_MIRIMAGE_F1130Wexp1_rate.fits ...
2020-10-14 14:22:43,464 - stpipe.Image2Pipeline.bkg_subtract - INFO - Step bkg_subtract running with args (<ImageModel(1024, 1032) from det_image_seq4_MIRIMAGE_F1130Wexp1_rate.fits>, ['IMA_background/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits']).
2020-10-14 14:22:43,465 - stpipe.Image2Pipeline.bkg_subtract - INFO - Step bkg_subtract parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'bsub', 'search_output_file': True, 'input_dir': '', 'sigma': 3.0, 'maxiters': None}
2020-10-14 14:22:43,841 - stpipe.Image2Pipeline.bkg_subtract - INFO - Step bkg_subtract done
2020-10-14 14:22:43,904 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs running with args (<ImageModel(1024, 1032) from det_image_seq4_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:43,905 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'assign_wcs', 'search_output_file': True, 'input_dir': '', 'slit_y_low': -0.55, 'slit_y_high': 0.55}
2020-10-14 14:22:44,036 - stpipe.Image2Pipeline.assign_wcs - INFO - Created a MIRI mir_image pipeline with references {'distortion': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_distortion_0028.asdf', 'filteroffset': '/Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_filteroffset_0005.asdf', 'specwcs': None, 'regions': None, 'wavelengthrange': None, 'camera': None, 'collimator': None, 'disperser': None, 'fore': None, 'fpa': None, 'msa': None, 'ote': None, 'ifupost': None, 'ifufore': None, 'ifuslicer': None}
2020-10-14 14:22:44,067 - stpipe.Image2Pipeline.assign_wcs - INFO - Update S_REGION to POLYGON ICRS 0.021816705 -0.019412262 0.024284311 0.011774907 359.993225201 0.014347561 359.990418134 -0.016853309
2020-10-14 14:22:44,068 - stpipe.Image2Pipeline.assign_wcs - INFO - assign_wcs updated S_REGION to POLYGON ICRS 0.021816705 -0.019412262 0.024284311 0.011774907 359.993225201 0.014347561 359.990418134 -0.016853309
2020-10-14 14:22:44,069 - stpipe.Image2Pipeline.assign_wcs - INFO - COMPLETED assign_wcs
2020-10-14 14:22:44,073 - stpipe.Image2Pipeline.assign_wcs - INFO - Step assign_wcs done
2020-10-14 14:22:44,135 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field running with args (<ImageModel(1024, 1032) from det_image_seq4_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:44,137 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'flat_field', 'search_output_file': True, 'input_dir': '', 'save_interpolated_flat': False, 'user_supplied_flat': None, 'inverse': False}
2020-10-14 14:22:44,195 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword CDP_PARTIAL_DATA does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:44,196 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword CDP_LOW_QUAL does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:44,196 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword CDP_UNRELIABLE_ERROR does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:44,203 - stpipe.Image2Pipeline.flat_field - WARNING - Keyword DIFF_PATTERN does not correspond to an existing DQ mnemonic, so will be ignored
2020-10-14 14:22:44,296 - stpipe.Image2Pipeline.flat_field - INFO - Step flat_field done
2020-10-14 14:22:44,368 - stpipe.Image2Pipeline.photom - INFO - Step photom running with args (<ImageModel(1024, 1032) from det_image_seq4_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:44,369 - stpipe.Image2Pipeline.photom - INFO - Step photom parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': False, 'skip': False, 'suffix': 'photom', 'search_output_file': True, 'input_dir': '', 'inverse': False, 'source_type': None}
2020-10-14 14:22:44,381 - stpipe.Image2Pipeline.photom - INFO - Using photom reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_photom_0044.fits
2020-10-14 14:22:44,382 - stpipe.Image2Pipeline.photom - INFO - Using area reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_area_0004.fits
2020-10-14 14:22:44,453 - stpipe.Image2Pipeline.photom - INFO - Using instrument: MIRI
2020-10-14 14:22:44,453 - stpipe.Image2Pipeline.photom - INFO - detector: MIRIMAGE
2020-10-14 14:22:44,454 - stpipe.Image2Pipeline.photom - INFO - exp_type: MIR_IMAGE
2020-10-14 14:22:44,455 - stpipe.Image2Pipeline.photom - INFO - filter: F1130W
2020-10-14 14:22:44,481 - stpipe.Image2Pipeline.photom - INFO - Pixel area map copied to output.
2020-10-14 14:22:44,483 - stpipe.Image2Pipeline.photom - INFO - subarray: FULL
2020-10-14 14:22:44,484 - stpipe.Image2Pipeline.photom - INFO - PHOTMJSR value: 2.44947
2020-10-14 14:22:44,492 - stpipe.Image2Pipeline.photom - INFO - Step photom done
2020-10-14 14:22:44,560 - stpipe.Image2Pipeline.resample - INFO - Step resample running with args (<ImageModel(1024, 1032) from det_image_seq4_MIRIMAGE_F1130Wexp1_rate.fits>,).
2020-10-14 14:22:44,561 - stpipe.Image2Pipeline.resample - INFO - Step resample parameters are: {'pre_hooks': [], 'post_hooks': [], 'output_file': None, 'output_dir': None, 'output_ext': '.fits', 'output_use_model': False, 'output_use_index': True, 'save_results': True, 'skip': False, 'suffix': 'i2d', 'search_output_file': True, 'input_dir': '', 'pixfrac': 1.0, 'kernel': 'square', 'fillval': 'INDEF', 'weight_type': 'exptime', 'single': False, 'blendheaders': False}
2020-10-14 14:22:44,578 - stpipe.Image2Pipeline.resample - INFO - Drizpars reference file: /Users/patrickkavanagh/crds_mirror/references/jwst/miri/jwst_miri_drizpars_0001.fits
2020-10-14 14:22:45,150 - stpipe.Image2Pipeline.resample - INFO - Drizzling (1024, 1032) --> (1023, 1023)
2020-10-14 14:22:45,582 - stpipe.Image2Pipeline.resample - INFO - Update S_REGION to POLYGON ICRS 0.021764821 -0.019608104 0.024515051 0.011731407 359.993144874 0.014484328 359.990394643 -0.016855183
2020-10-14 14:22:45,706 - stpipe.Image2Pipeline.resample - INFO - Saved model in demo_output/my_galaxy_dither4_i2d.fits
2020-10-14 14:22:45,706 - stpipe.Image2Pipeline.resample - INFO - Step resample done
2020-10-14 14:22:45,707 - stpipe.Image2Pipeline - INFO - Finished processing product my_galaxy_dither4
2020-10-14 14:22:45,708 - stpipe.Image2Pipeline - INFO - ... ending calwebb_image2
2020-10-14 14:22:45,944 - stpipe.Image2Pipeline - INFO - Saved model in demo_output/my_galaxy_dither1_cal.fits
2020-10-14 14:22:46,178 - stpipe.Image2Pipeline - INFO - Saved model in demo_output/my_galaxy_dither2_cal.fits
2020-10-14 14:22:46,406 - stpipe.Image2Pipeline - INFO - Saved model in demo_output/my_galaxy_dither3_cal.fits
2020-10-14 14:22:46,643 - stpipe.Image2Pipeline - INFO - Saved model in demo_output/my_galaxy_dither4_cal.fits
2020-10-14 14:22:46,644 - stpipe.Image2Pipeline - INFO - Step Image2Pipeline done
We can plot the before (rate) and after (cal) images for the first dither position
# open the input image as a jwst data model
with datamodels.open(my_science_files[0]) as in_dm:
fig, axs = plt.subplots(1, 2, figsize=(14, 7), sharey=True)
axs[0].imshow(in_dm.data, cmap='jet', interpolation='nearest', origin='lower', norm=LogNorm(vmin=10, vmax=1000))
axs[0].annotate('DMS Level 2A (rate)', xy=(0.0, 1.02), xycoords='axes fraction', fontsize=12, fontweight='bold', color='k')
axs[0].set_facecolor('black')
axs[1].imshow(dm_cont[0].data, cmap='jet', interpolation='nearest', origin='lower', norm=LogNorm(vmin=10, vmax=1000))
axs[1].annotate('DMS Level 2B (cal)', xy=(0.0, 1.02), xycoords='axes fraction', fontsize=12, fontweight='bold', color='k')
axs[1].set_facecolor('black')
plt.tight_layout()
plt.show()
2020-10-14 14:22:52,098 - stpipe - WARNING - /Users/patrickkavanagh/anaconda3/anaconda3/envs/jwst7.6/lib/python3.8/site-packages/ipykernel/ipkernel.py:287: DeprecationWarning: `should_run_async` will not call `transform_cell` automatically in the future. Please pass the result to `transformed_cell` argument and any exception that happen during thetransform in `preprocessing_exc_tuple` in IPython 7.17 and above.
and should_run_async(code)
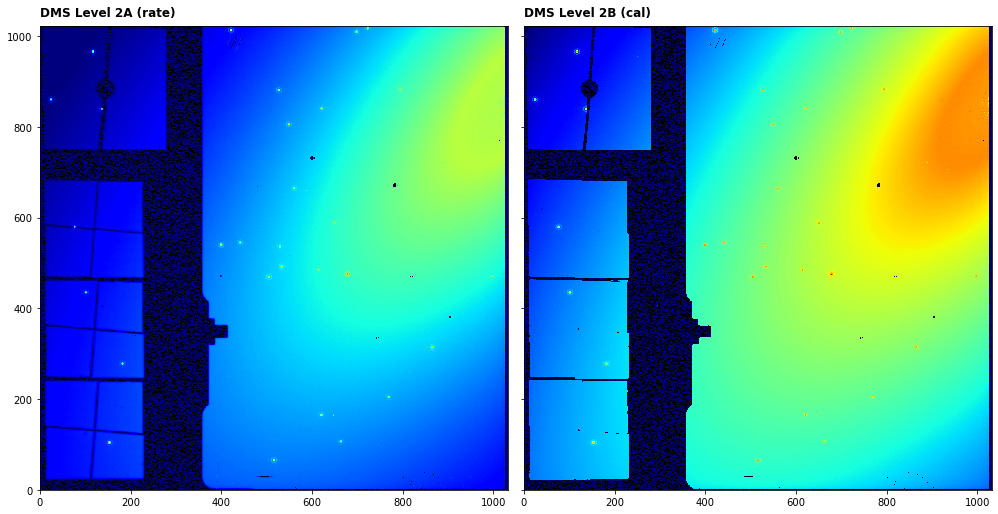
Command line¶
To achieve the same result from the command line there are a couple of options. However, one must still generate the association file. In this case, it is best to copy the template above into a text file and save it to my_galaxy_lvl2_asn.json
. The content is printed here for convenience.
{
"asn_type": "image2",
"asn_rule": "DMSLevel2bBase",
"version_id": null,
"code_version": "0.13.7",
"degraded_status": "No known degraded exposures in association.",
"program": "noprogram",
"products": [
{
"name": "my_galaxy_dither1",
"members": [
{
"expname": "IMA_science/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "science"
},
{
"expname": "IMA_background/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "background"
}
]
},
{
"name": "my_galaxy_dither2",
"members": [
{
"expname": "IMA_science/det_image_seq2_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "science"
},
{
"expname": "IMA_background/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "background"
}
]
},
{
"name": "my_galaxy_dither3",
"members": [
{
"expname": "IMA_science/det_image_seq3_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "science"
},
{
"expname": "IMA_background/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "background"
}
]
},
{
"name": "my_galaxy_dither4",
"members": [
{
"expname": "IMA_science/det_image_seq4_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "science"
},
{
"expname": "IMA_background/det_image_seq1_MIRIMAGE_F1130Wexp1_rate.fits",
"exptype": "background"
}
]
}
],
"asn_pool": "my_galaxy_pool"
}
Option 1:
Run the Image2Pipeline
class using the strun
command:
mkdir demo_output
strun jwst.pipeline.Image2Pipeline my_galaxy_lvl2_asn.json --output_dir demo_output
This will produce the same output file in the user-defined --output_dir
Option 2:
Collect the pipeline configuration files in your working directory (if they are not already there) using collect_pipeline_configs
and then run the Image2Pipeline
using the strun
command with the associated calwebb_image2.cfg
file. This option is a little more flexible as one can create edit the cfg files, use them again, etc.
mkdir demo_output
collect_pipeline_cfgs cfgs/
strun cfgs/calwebb_image2.cfg my_galaxy_lvl2_asn.json --output_dir demo_output
This will produce the same output file in the user-defined --output_dir